Advanced Map Customizations
This page gives an overview of possible customizations of the Map facet, among which:
- Customize Google Maps API loading, or localize your map
- Change the map type
- Turn off specific map controls
- Reposition the map controls
- Customize map gestures behavior
- Customize the map style/design
- Set a custom zoom level or location/center, or Restrict the map viewport
- Customize the marker pins
- Customize the Proximity facet marker pin
- Customize or overwrite the marker infowindow content
- Customize marker clustering behavior
- Customize Overlapping Marker Spiderfier behavior
- How to use advanced map marker hooks and functions
- How to add a Map facet to single posts or pages
Customize Google Maps API loading
Add URL parameters
When FacetWP loads a Google map, the script loading URL contains several specific URL parameters that determine which Google Map libraries are loaded and what your Google Maps API key is. The URL looks like this:
https://maps.googleapis.com/maps/api/js?libraries=places&key=YOUR_API_KEY&callback=Function.prototype
As is standard in URLs, all parameters must be preceded by an ampersand (&
) character, except the first one directly following the ?
. Note that the order of the parameters is not relevant, and that the callback
parameter is required, even if there is no actual callback function (which is why FacetWP uses callback=Function.prototype
).
Using the facetwp_gmaps_url
filter, you can filter the whole URL and change these parameters, or pass additional ones to the Google Maps API. See the localization code snippet below for an example.
Localize your map
By default, the Google Maps API will attempt to automatically load the most appropriate language and region based on the user’s location or browser settings. However, if you want to explicitly set a fixed region and/or language for the map, you can use the region
and language
parameters.
The region parameter allows you to localize the behavior and features of the map, based on the country or region set. The language parameter parameters lets you translate the map’s user interface.
The following example sets the map’s region to The Netherlands, and the language to Dutch:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_gmaps_url', function( $url) { $url = $url . '®ion=NL&language=nl'; // Adds the 'region' and 'language' parameters to the end of the URL return $url; } );
Use your own Google Maps API script
If your theme or another plugin already loads a Google Maps API script, you’ll have to choose which script to use, as loading the Google Maps API multiple times on the page will lead to errors.
If you choose to use the script that is already there, make sure it loads the Places library that FacetWP needs (with the libraries=places
parameter). To remove FacetWP’s Google Maps API script, you can use the facetwp_assets hook like this:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_assets', function( $assets ) { unset( $assets['gmaps'] ); return $assets; });
Change the map type
Google maps can be displayed in four different basic map types:
roadmap
displays the default road map view.satellite
displays Google Earth satellite images.hybrid
displays a mixture of normal and satellite views.terrain
displays a physical map based on terrain information.
The default map type is roadmap
, which the user can change to the other map types with a Map Type Control (see below) in the left top corner of the map. You can set any of the four map types as default. The following code sets the map type to terrain
:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function ( $args ) { $args['init']['mapTypeId'] = 'terrain'; // valid options are: roadmap, satellite, hybrid, terrain return $args; });
Turn off the map controls
Google maps display several UI elements to allow user interaction with the map. These elements are known as “controls”.
The default controls can be individually, or all removed with the following code:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function ( $args ) { $args['init']['zoomControl'] = false; // +- zoom control $args['init']['mapTypeControl'] = false; // roadmap / satellite toggle $args['init']['streetViewControl'] = false; // street view / yellow man icon $args['init']['fullscreenControl'] = false; // full screen icon /** this overwrites all 4 lines above and will disable ALL of the default ui icons instead of the individual icons above */ $args['init']['disableDefaultUI'] = true; // disable the default ui return $args; } );
Reposition the map controls
Besides turning them off, you can also reposition the map controls, with the code below.
You have to reposition each control separately. Use the control names from the previous code example, or check the controls documentation.
It does not work to pass the documented control positions directly to the Map facet. You have to pass the number of which it is an alias:
TOP_LEFT: 1, TOP_CENTER: 2, TOP: 2, TOP_RIGHT: 3, LEFT_CENTER: 4, LEFT_TOP: 5, LEFT: 5, LEFT_BOTTOM: 6, RIGHT_TOP: 7, RIGHT: 7, RIGHT_CENTER: 8, RIGHT_BOTTOM: 9, BOTTOM_LEFT: 10, BOTTOM_CENTER: 11, BOTTOM: 11, BOTTOM_RIGHT: 12, CENTER: 13
The following example moves the “Zoom Control” (the plus-minus buttons) to the left bottom position:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function ( $args ) { $args['init']['zoomControl'] = true; $args['init']['zoomControlOptions']['position'] = 6; // 6 = LEFT_BOTTOM return $args; } );
Customize map gestures behavior
To influence how scrolling and touch events influence zooming and panning the map, you can use the gestureHandling map option.
If you set it to cooperative
, scroll events and one-finger touch gestures scroll the page, and do not zoom or pan the map. Two-finger touch gestures will still pan and zoom the map.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function ( $args ) { $args['init']['gestureHandling'] = 'cooperative'; // Default: 'auto' return $args; } );
See this Google Map section on gestureHandling for code examples and demo of all options.
Use a custom map style / design
The Map facet type offers four alternative styles besides the default look, which you can choose with the “Map design” setting.
If these styles are not what you are looking for, it is possible to design your own map style or download a pre-made style from a map style library. With a custom map style, you can not only change the color scheme, but also how and if map features like roads, administrative areas or points of interest are displayed, or not:
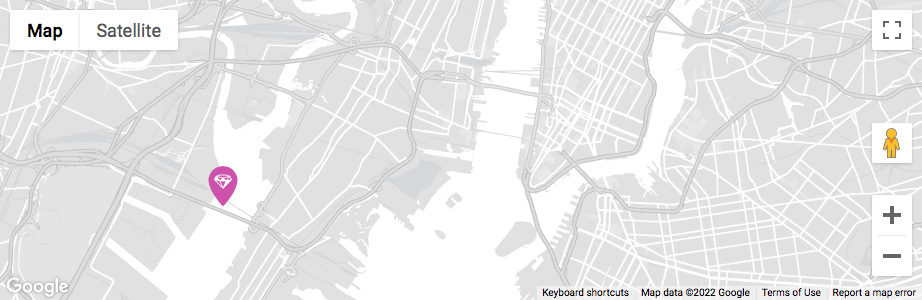
Two websites where you can build your own or download a pre-made custom map style are:
To use a custom map style:
- Build or choose the map style in one of the mentioned websites;
- Copy or download the resulting JSON array (which begins and ends with square brackets);
- Replace the JSON array in the code example below, between the single quotes, with your copied / downloaded JSON array;
- Add the code to your (child) theme’s function.php.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { $args['init']['styles'] = json_decode( '[{"featureType":"administrative","elementType":"labels.text.fill","stylers":[{"color":"#444444"}]},{"featureType":"landscape","elementType":"all","stylers":[{"color":"#f2f2f2"}]},{"featureType":"poi","elementType":"all","stylers":[{"visibility":"off"}]},{"featureType":"road","elementType":"all","stylers":[{"saturation":-100},{"lightness":45}]},{"featureType":"road.highway","elementType":"all","stylers":[{"visibility":"simplified"}]},{"featureType":"road.arterial","elementType":"labels.icon","stylers":[{"visibility":"off"}]},{"featureType":"transit","elementType":"all","stylers":[{"visibility":"off"}]},{"featureType":"water","elementType":"all","stylers":[{"color":"#46bcec"},{"visibility":"on"}]}]' ); return $args; } );
Alternatively, you can download and install the SnazzyMaps WordPress plugin and select a pre-made custom style. If you connect an API key in the settings, you can also select your own custom styles made on the SnazzyMaps website.
Set a custom zoom level or location/center
To determine the map center and correct zoom level, the map uses Google Maps’ fitBounds
function. On every refresh, the map automatically pans to the center of all found markers, and zooms to the zoom level where all found markers fit within the bounds of the map. There is no easy way to change the zoom level that fitBounds
ends up using after a page load or refresh.
The Map facet does have a setting called “Fallback lat / lng / zoom” where you can enter a custom center (lat/lng) and/or zoom level. These values are only used as a fallback, when no results/markers are found. In all other situations, these settings have no effect because the map uses the fitBounds
function.
However, with a bit of custom code, this behaviour can be overwritten, so you can actually use the “Fallback lat / lng / zoom” setting to set a custom center and zoom level on initial page load, or on every facet refresh.
First, set a custom lat/lng and zoom level in the Map facet settings.
Then add one of the following two code snippets to your (child) theme’s function.php:
Set a custom zoom level and location/center on initial page load only
To disable fitBounds
and force the custom lat/lng and zoom settings on the initial page load only:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php add_action( 'facetwp_scripts', function() { ?> <script> document.addEventListener('facetwp-refresh', function() { if ('undefined' !== typeof FWP && 'undefined' !== typeof FWP.hooks) { FWP.hooks.addFilter('facetwp_map/fit_bounds', function(fit_bounds) { return FWP.loaded; // force the custom lat/lng/zoom only on initial page load }); } }); </script> <?php }, 100 );
Set a custom zoom level and location/center on every refresh
To disable fitBounds
and force the custom lat/lng and zoom settings on initial page load and every facet refresh:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php add_action( 'facetwp_scripts', function() { ?> <script> document.addEventListener('facetwp-refresh', function() { if ('undefined' !== typeof FWP && 'undefined' !== typeof FWP.hooks) { FWP.hooks.addFilter('facetwp_map/fit_bounds', function(fit_bounds) { return false; // force the custom lat/lng/zoom on every refresh }); } }); </script> <?php }, 100 );
If you combine one of the above code snippets with Google Map’s restriction
parameter, you can also restrict the map’s viewport to specified bounds.
To only set a custom zoom level on page load, see the two sections below:
Set a custom zoom level on initial page load only
If you want to only set a custom zoom level, and leave the lat/lng determined by the fitBounds
function (as explained above) intact, the above solutions that use the “Fallback lat / lng / zoom” setting, will not work. This is because if you leave the fallback lat/lng empty, it will default to 0,0
and your map will load with that location as its center.
The following code will only set a custom zoom level, only on initial page load:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'facetwp_scripts', function() { ?> <script> function setCustomZoom() { google.maps.event.addListenerOnce(FWP_MAP.map, 'tilesloaded', function(){ FWP_MAP.map.setZoom(5); // Custom zoom level, set only on first page load. document.removeEventListener('facetwp-maps-loaded', setCustomZoom); }); } document.addEventListener('facetwp-refresh', function() { if( ! FWP.loaded ) { // On first page load only. document.addEventListener('facetwp-maps-loaded', setCustomZoom); } }); </script> <?php }, 100 );
Set a custom zoom level on every refresh
The following code will (re)set a custom zoom level on every facet refresh of the map:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'facetwp_scripts', function() { ?> <script> document.addEventListener('facetwp-maps-loaded', function() { google.maps.event.addListenerOnce(FWP_MAP.map, 'tilesloaded', function(){ FWP_MAP.map.setZoom(5); // Custom zoom level, set on each refresh. }); }); </script> <?php }, 100 );
Restrict the map viewport
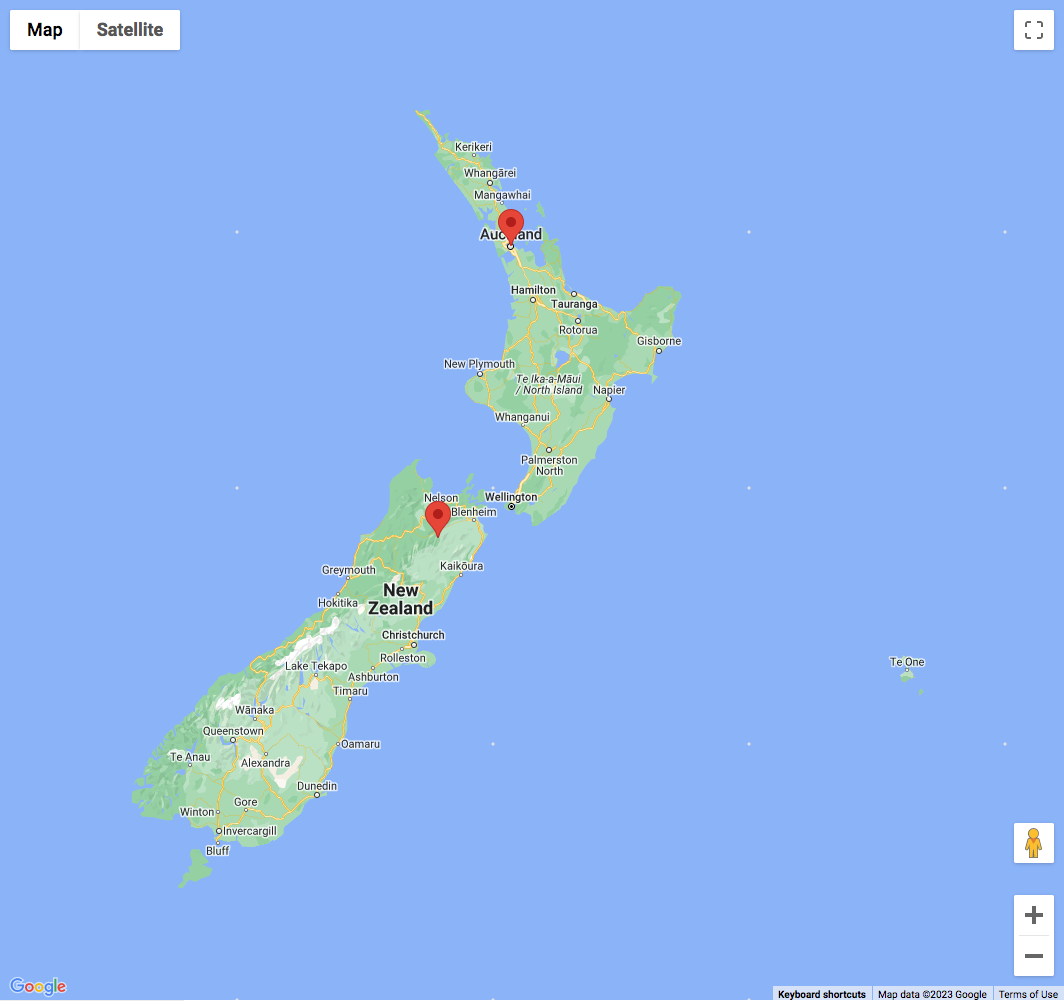
Instead of using the “Fallback lat/lng/zoom” in the Map facet’s settings, it is also possible to restrict the map’s viewport with Google Map’s restriction parameter. With this parameter set, the user cannot pan or zoom out beyond the specified viewport bounds. Manually zooming in is still possible, within the range set with the Zoom min/max setting.
The following code example restricts the map’s viewport to the bounds of New Zealand, and sets the center to Auckland.
To make this code work, it must be combined with the above snippet to disable the fitBounds function on every refresh, otherwise the map will still try to pan and scroll when using facets, which will lead to unexpected behavior.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { // Auckland New Zealand $args['init']['center'] = [ 'lat' => -36.8598108, 'lng' => 174.2117363 ]; // New Zealand bounds $args['init']['restriction'] = [ 'latLngBounds' => [ 'north' => -34.36, 'south' => -47.35, 'west' => 166.28, 'east' => -175.81 ], 'strictBounds' => false ]; $args['init']['zoom'] = 6; // Is used if no Fallback lat/lng and zoom are set in the Map facet settings. See explanation below. return $args; } );
There are a few settings that determine the zoom level that is used when the map loads with a restricted viewport: the zoom
parameter in line 20 of above code, and the Fallback lat/lng/zoom setting in the Map facet settings. They influence each other as follows.
If you want the map to use the exact restricted viewport when it loads, don’t use the zoom
parameter and leave the fallback zoom setting empty. Or, if you use any or both of them, make sure the zoom level is lower (zoomed out more) than the equivalent of the restricted viewport: the map will never zoom out more than the restricted viewport.
To zoom into the restricted viewport on page load, you can set a higher zoom level in one or both of these settings. Be aware that they override each other as follows:
- Without the
zoom
parameter set, a fallback zoom level will determine the zoom parameter, also if no fallback lat/lng is set. - With the
zoom
parameter set, a fallback zoom level will override the zoom parameter, but only if a fallback lat/lng is also set.
Customize the marker pins
The simplest way to change the marker pin images is with the following code. Make sure to use a transparent PNG or SVG.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_marker_args', function( $args, $post_id ) { $args['icon'] = get_stylesheet_directory_uri() . '/assets/images/marker.png'; // set your theme image path here return $args; }, 10, 2 );
For more flexibility, you could alternatively use arrays to mimic Google Map API’s JS objects:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_marker_args', function( $args, $post_id ) { $args['icon'] = [ 'url' => get_stylesheet_directory_uri() . '/assets/images/marker.png', // set your theme image path here 'scaledSize' => [ 'width' => 16, 'height' => 16 ] ]; return $args; }, 10, 2 );
This also gives you access to other properties to fine-tune the position of the marker icon, like anchor
and labelOrigin
(to position marker labels). See Google maps documentation on the google.maps.Icon interface for an overview of available properties.
Customize the marker pins for selected posts only
The following example is the same as the previous one, but changes the marker pins only for specific posts:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_marker_args', function( $args, $post_id ) { $myposts_with_custom_markers = array(101, 102, 103); // array with the post id's of posts that need a custom marker if ( in_array( $post_id, $myposts_with_custom_markers ) ) { $args['icon'] = [ 'url' => get_stylesheet_directory_uri() . '/assets/images/marker.png', // set your theme image path here 'scaledSize' => [ 'width' => 16, 'height' => 16 ] ]; } return $args; }, 10, 2 );
Customize the marker pins for each post category or term
You could select posts based on any post property available through the $post_id
parameter. An example would be to give each post category/term a different marker pin, using has_category() or has_term():
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_marker_args', function( $args, $post_id ) { if (has_term( 'my-term-slug', 'my-taxonomy', $post_id)) { // replace with your term slug and taxonomy $args['icon'] = [ 'url' => get_stylesheet_directory_uri() . '/assets/images/marker.png', // set your theme image path here 'scaledSize' => [ 'width' => 16, 'height' => 16 ] ]; } return $args; }, 10, 2 );
Use a (featured) image as marker pin
With the following code, you can set an image (for example the post’s featured image) as the marker pin. This makes it possible to display for example company logos or user portraits on the map:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_marker_args', function( $args, $post_id ) { if ( has_post_thumbnail( $post_id ) ) { $member_photo = get_the_post_thumbnail_url( $post_id, 'post-thumbnail' ); // Set your desired wp image size and adapt scaledSize below } else { $member_photo = get_home_url() . '/wp-content/uploads/default-member-photo.jpg'; // Use a fall-back image if a post does not have a featured image uploaded } $args['icon'] = [ 'url' => $member_photo, 'scaledSize' => [ 'width' => 50, 'height' => 50 ] ]; return $args; }, 10, 2 );
Adapt the scaledSize
argument to the desired marker image’s width and height. To avoid image distortion, set a WP image size with the same size or proportions in the second parameter of the get_the_post_thumbnail_url() function. The image size defaults to post-thumbnail
, but you can use any image size set in Settings > Media
or your theme’s settings. Or you can create your own image size with add_image_size(). For more info about how image sizes in WordPress work, see this tutorial section.
Style marker pin images
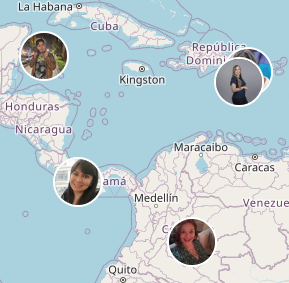
If you try to style the marker pin images (set with above code), you’ll notice that they are hard to target with CSS, as they do not have a specific class.
A solution is to let your CSS target <img>
tags within the map that have a src
attribute that starts with (^=
) your image directory, in this example /wp-content/uploads/
. Make sure that your fallback image is also in the same directory.
The following snippet adds CSS that makes the marker pin images circular, with a 2px
white border:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_head', function() { ?> <style> .facetwp-type-map img[src^="https://mysite.com/wp-content/uploads/"] { border-radius: 50%; /* circular */ border: 2px solid #fff !important; /* 2px white border */ box-sizing: border-box; /* make sure the border is within the set image dimension */ } </style> <?php }, 100 );
Change the marker pins when an infoWindow is open
It is possible the change the (custom) marker icon when an infoWindow is clicked open. See the example below for how to accomplish that.
Add a marker label
Using the facetwp_map_marker_args
filter, you can pass properties of the Google Maps MarkerLabel interface. This makes it possible to add a marker label above, below, or beside your marker.
The following example adds a marker label with the post title as text. Optionally, you can set a few other properties like fontSize
and fontWeight
.
The label will display at the exact origin of your marker, so with a marker already there it will be centered on top of the marker. To position the label relative to the marker, you can pass a custom class (in this example label-position
):
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_marker_args', function( $args, $post_id ) { $labeltext = esc_html( get_the_title( $post_id ) ); // Fetch the post title. $args['label'] = [ 'text' => $labeltext, 'className' => 'label-position', // A custom class. // optional: 'color' => 'red', 'fontSize' => '40px', 'fontWeight' => 'bold', 'fontFamily' => 'Courier' ]; return $args; }, 10, 2 );
Using this custom class, the label can now be relatively positioned with a few lines of CSS. Note that to position the label above the marker, you can use a negative value for top
:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_head', function() { ?> <style> .facetwp-type-map .label-position { position: relative; top: -40px; /* Use a negative value to position the label above the marker. And you can use 'bottom', 'left' and 'right'. */ } </style> <?php } );
As an alternative to positioning the label with CSS, if you are using custom markers pins, you can use the labelOrigin
property to position the label. To position the label above the marker, use a negative value for y
:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_marker_args', function( $args, $post_id ) { $args['icon'] = [ 'url' => get_stylesheet_directory_uri() . '/assets/images/marker.png', // Set your theme image path here. 'scaledSize' => [ 'width' => 16, 'height' => 16 ], 'labelOrigin' => [ 'x' => 0, // You can use negative values. 'y' => -50 // Use a negative value to position the label above the marker. ] ]; return $args; }, 10, 2 );
Add a label and remove the marker pin
If you want to add a marker label, while removing the marker icon itself, you can use an empty icon
argument (with a space between the quotes) to unset the marker icons:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_marker_args', function( $args, $post_id ) { $labeltext = esc_html( get_the_title( $post_id ) ); // Fetch the post title. $args['label'] = [ 'text' => $labeltext, 'className' => 'label-position', // A custom class. // optional: 'color' => 'red', 'fontSize' => '40px', 'fontWeight' => 'bold', 'fontFamily' => 'Courier' ]; $args['icon'] = ' '; // Removes the marker icons. Note: for this to work, there needs to be a space between the quotes. return $args; }, 10, 2 );
Make (some) markers unclickable
If you need (some) markers to be unclickable, you can set their clickable
marker argument to false
with the facetwp_map_marker_args
hook. The hook has access to the post ID of each marker it runs for, so you can check that post ID against an array of post IDs for which the markers must be unclickable:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_marker_args', function( $args, $post_id ) { $disable_marker_click = array( 5648, 5678 ); // Create an array with the post IDs of posts with unclickable markers if ( in_array( $post_id, $disable_marker_click ) ) { $args['clickable'] = false; // Make this marker unclickable } return $args; }, 10, 2 );
Another approach is to use the $post_id
to check the value of a custom field. For example, you could create an is_clickable
True/False field with Advanced Custom Fields and get the value of that field for each marker’s post ID:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_marker_args', function( $args, $post_id ) { if ( get_field( 'is_clickable', $post_id ) === false ) { $args['clickable'] = false; // Make this marker unclickable } return $args; }, 10, 2 );
Display a circle or other shape around clicked markers
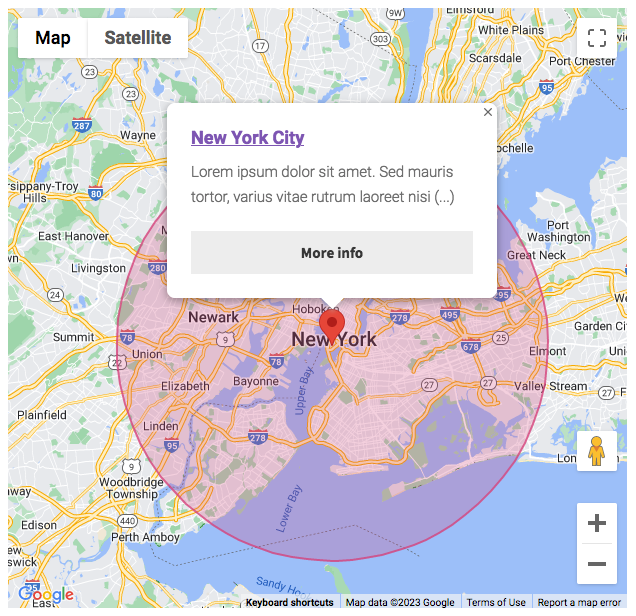
The following code draws a circle around a marker when it is clicked. Note that instead of a circle, this could be any other type of shape/polygon supported by the Google Maps API.
You can customize the circle’s fill color and opacity, and its stroke color, opacity and weight. The circle’s size is determined by the radius
value (in meters) set in line 22.
Clicking another marker will clear the previous circle. The code (optionally) also removes the circle when any marker’s infowindow is closed, and/or when you click anywhere on the map.
If you want to apply the code only to certain markers, you can use the clicked marker’s post_id
to write a condition.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'facetwp_scripts', function() { ?> <script> (function() { FWP.hooks.addAction('facetwp_map/marker/click', function(marker) { // Clear existing circle if it exists if ( 'undefined' !== typeof FWP_MAP.circle ) { FWP_MAP.circle.setMap(null); // Clear circle from map FWP_MAP.circle = null; // Remove circle instance entirely } // let post_id = marker.post_id; // The marker's post_id could be used to apply this code only to certain markers. let lat = marker.position.lat(); let lng = marker.position.lng(); FWP_MAP.circle = new google.maps.Circle({ center: { lat: lat, lng: lng }, radius: 25000, // Circle radius in meters fillColor: "#D93F7C", // Circle coloring fillOpacity: 0.25, // Circle opacity strokeColor: "#D93F7C", // Circle outline color strokeOpacity: 0.8, // Circle outline color strokeWeight: 2, // Circle outline thickness map: FWP_MAP.map }); // Optional: when an infoWindow is closed, clear the circle from the map google.maps.event.addListener(FWP_MAP.infoWindow, 'closeclick', function() { FWP_MAP.circle.setMap(null); }); // Optional: when the map is clicked anywhere, clear the circle from map google.maps.event.addListener(FWP_MAP.map, 'click', function(event) { FWP_MAP.circle.setMap(null); }); }); })(); </script> <?php }, 100 );
To display the Proximity facet radius as a circle on the map, see the example below.
Customize the Proximity facet marker pin
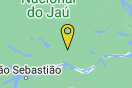
If you are using the map with a Proximity facet, when a location is entered, a yellow location marker is displayed on the map.
With the following code, you can change the proximity marker pin’s path, color, opacity, scale, and anchor. The code example shows the default icon settings. All of these settings need to have a value.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_proximity_marker_args', function( $args ) { $args['icon'] = [ 'path' => 'M8,0C3.582,0,0,3.582,0,8s8,24,8,24s8-19.582,8-24S12.418,0,8,0z M8,12c-2.209,0-4-1.791-4-4 s1.791-4,4-4s4,1.791,4,4S10.209,12,8,12z', 'fillColor' => 'gold', 'fillOpacity' => 0.8, 'scale' => 0.8, 'anchor' => [ 'x' => 8.5, 'y' => 32 ] ]; return $args; }, 10 );
You can also replace the default proximity marker pin with a custom marker image, with the following code. Make sure to use a transparent PNG or SVG.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_proximity_marker_args', function( $args ) { $args['icon'] = get_stylesheet_directory_uri() . '/assets/images/marker.png'; // set your theme image path here return $args; }, 10 );
For more flexibility, you could alternatively use arrays to mimic Google Map API’s JS objects:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_proximity_marker_args', function( $args ) { $args['icon'] = [ 'url' => get_stylesheet_directory_uri() . '/assets/images/marker.png', // set your theme image path here 'scaledSize' => [ 'width' => 16, 'height' => 16 ] ]; return $args; }, 10 );
This also gives you access to other properties to fine-tune the position of the marker icon, like anchor
and labelOrigin
(to position marker labels). See Google maps documentation on the google.maps.Icon interface for an overview of available properties.
Disable the Proximity facet marker pin
If you want to completely disable the Proximity marker pin, you can use this hook:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_proximity_marker_args', '__return_false' );
If you have enabled marker clustering, you can also exclude the Proximity marker pin from the clustering behavior.
Display the Proximity radius as a circle on the map
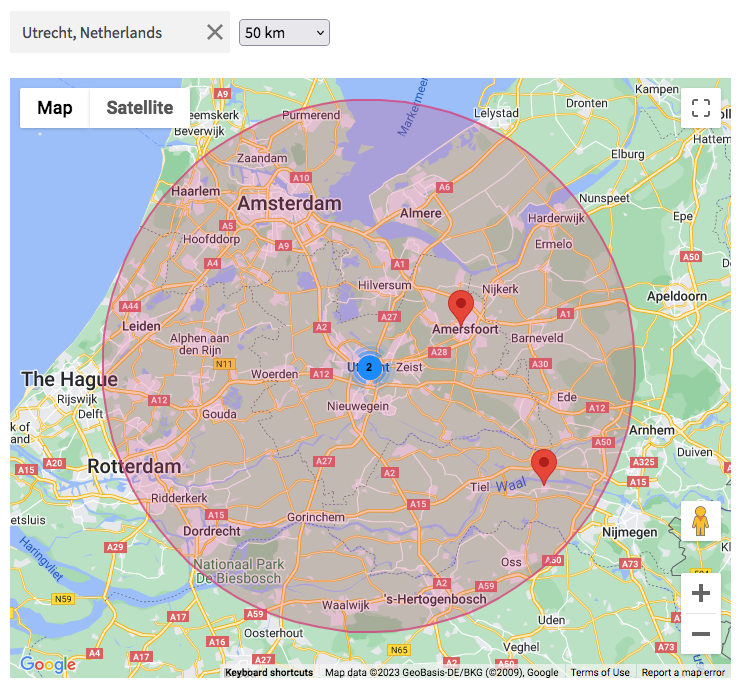
The following code draws a circle shape on the map each time a location is entered in the Proximity facet. The circle’s radius will adapt automatically to the radius set in the Proximity facet.
You can customize the circle’s fill color and opacity, and its stroke color, opacity and weight. Make sure to replace my_proximity_facet
with the name of your proximity facet in line 14, and set the correct number of units (kilometers or miles) in line 17, depending on the “Units of measurement” setting you have used.
Be aware that the map’s default fitBounds
behavior will make the map zoom into the found locations when the Proximity facet is used. The circle’s edge will then end up outside or around the viewport of the map. If you want the entire circle to be always visible, you could set a maximum zoom level in the map’s Zoom min / max setting, or make the circle smaller than the Proximity radius (which will possibly cause some markers to be outside the circle). In some situations, it may be an idea to disable the fitBounds
behavior entirely and set a custom zoom level and/or center.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'facetwp_scripts', function() { ?> <script type="text/javascript"> (function() { document.addEventListener('facetwp-loaded', function() { // Clear existing circle if it exists if ( 'undefined' !== typeof FWP_MAP.circle ) { FWP_MAP.circle.setMap(null); // Clear circle from map FWP_MAP.circle = null; // Remove circle instance entirely } let proximityfacet = FWP.facets['my_proximity_facet']; // Replace "my_proximity_facet" with the name of your Proximity facet let lat = parseFloat(proximityfacet[0]); let lng = parseFloat(proximityfacet[1]); let radius = parseFloat(proximityfacet[2]) * 1000; // For Kilometers. Use 1609.344 if you have set the Unit of measurement setting to Miles FWP_MAP.circle = new google.maps.Circle({ center: { lat: lat, lng: lng }, radius: radius, // Circle radius in meters fillColor: "#D93F7C", // Circle coloring fillOpacity: 0.25, // Circle opacity strokeColor: "#D93F7C", // Circle outline color strokeOpacity: 0.8, // Circle outline color strokeWeight: 2, // Circle outline thickness map: FWP_MAP.map }); if (proximityfacet.length) { FWP_MAP.circle.setMap(FWP_MAP.map); } }); })(); </script> <?php }, 100 );
To display a circle (or other shape) around clicked markers, see the example above.
Customize or overwrite the marker infowindow content
The marker infowindow content can be created in the Map facet’s “Marker content” setting field.
If this field is too limiting, or if you can’t reach certain data with it, you can use the facetwp_map_marker_args
hook to output the marker infowindow content. Note that this will overwrite anything you have in the “Marker content” setting.
As $post_id
is available in this hook, you can create or overwrite the content conditionally, only for specific posts:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_marker_args', function( $args, $post_id ){ if ( $post_id == 5647 ) { // Optionally add a condition to check for specific post IDs $args['content'] = "my custom marker content"; // Create your custom marker infowindow content } return $args; }, 10, 2 );
One use case for this is if you use ACF Repeater fields to support multiple locations per post. With the “Marker content” field you can only get post data, but not the data for the separate locations within the repeater. This snippet shows how to get and output the repeater field data with the facetwp_map_marker_args
hook.
Marker clustering
If you have large numbers of markers in one area, you can enable the marker clustering option. Marker clustering combines markers of close proximity into clusters, simplifying the display of markers on the map.
The marker clusterer library used by FacetWP, and its options, methods and examples can be found here.
Set marker cluster to zoom in when clicking on it
The behaviour of the map after clicking on a marker cluster can be set to zoom into it with this code:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { if ( isset( $args['config']['cluster'] ) ) { $args['config']['cluster']['zoomOnClick'] = true; // default: false } return $args; } );
Set the maximum zoom level for a cluster to appear
The maximum zoom level that a marker can be part of a cluster can be set like this:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { if ( isset( $args['config']['cluster'] ) ) { $args['config']['cluster']['maxZoom'] = 10; // default: 15. Level must be between 1 and 20. } return $args; } );
Set the minimum number of markers in a cluster
The minimum number of markers to be in a cluster before the markers are hidden and a count is shown can be set like this:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { if ( isset( $args['config']['cluster'] ) ) { $args['config']['cluster']['minimumClusterSize'] = 5; // default: 2 } return $args; } );
Customize the marker cluster images
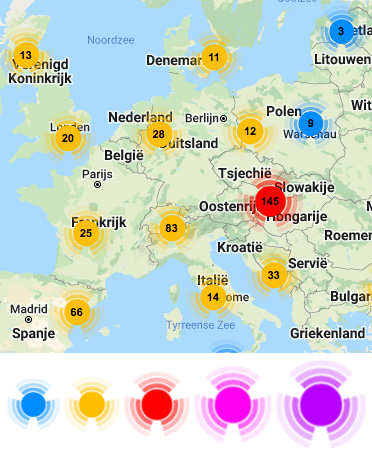
The marker clusterer library used by FacetWP comes with five PNG cluster icon images, in ascending specific sizes.
The cluster icons’ sizes and colors represent the number of markers in the cluster. Which of the five cluster icons is shown, is calculated based on the number of markers in that specific cluster. From the smallest to the largest icon, the conditions to show that icon are: <10
, <100
, <1000
, <10000
, and >=10000
markers.
With the two methods described below, it is possible to change the default cluster images and their sizes.
Using the second method, you can also change the icon number’s text size and text color (which is black by default).
Method 1: Using imagePath and imageExtension
To simply replace the five default marker cluster icons with your own icons, use the following code:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { if ( isset( $args['config']['cluster'] ) ) { $args['config']['cluster']['imagePath'] = get_stylesheet_directory_uri() . '/assets/img/googlemaps/m'; // set your own image directory in your theme here. Note: the /m at the end is not a directory, but the first part of the image names. $args['config']['cluster']['imageExtension'] = 'png'; // 'svg' will work too } return $args; } );
For this to work, you need to make a custom directory in your theme. In the above example, this directory would be /assets/img/googlemaps
. The /m
in the specified directory is the first part of the image name.
In this example, the five images need the following specific names and sizes to work:
m1.png (size: 53x53 px, default color: blue) m2.png (size: 56x56 px, default color: yellow) m3.png (size: 66x66 px, default color: red) m4.png (size: 78x78 px, default color: magenta) m5.png (size: 90x90 px, default color: purple)
Note: You can use SVGs instead of PNGs. Just change imageExtension
to 'svg'
.
Method 2: Using the styles array
If you need more control, instead of using the imagePath
and imageExtension
options, you can use the styles array. With this method you can not only set custom cluster images and sizes, but also change the cluster icons’ text color and text sizes.
The following example uses the default icon directory and icon sizes. It sets the cluster icon text (the number of markers in the cluster) to a size of 16px, and the color to #ffffff (white).
If you want to use custom icons and sizes, you can specify a directory in your theme with the 'url'
option, and place five images in it (see the previous example). Note that the /m
in the specified directory is not a directory but the first part of the image name. You can specify five custom icon sizes in the $clustericonsizes
array.
The two-dimensional styles
array needs five child arrays, for each of the five marker cluster icons, in ascending sizes:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $settings ) { $clustericonsizes = array( 53, 56, 66, 78, 90 ); // specify icon image sizes. These are the default values. for ( $i = 1; $i <= 5; $i ++ ) { $settings['config']['cluster']['styles'][] = [ 'url' => FACETWP_MAP_URL . '/assets/img/m' . $i . '.png', // directory, required. This is the default directory. The url becomes the CSS background-image url. 'width' => $clustericonsizes[ $i - 1 ], // required 'height' => $clustericonsizes[ $i - 1 ], // required 'textColor' => '#ffffff', // white, optional 'textSize' => 16 // 16px, optional // 'anchor' => [10,20], // Optional. Anchor position of the label text. By default label text is centered horizontally and vertically in the icon. [10,20] means 10px from the top of the icon (y) and 20px (x) from the left of the icon. Values need to be smaller than width and height of the icon to take effect. // 'iconAnchor' => [10,20], // Optional. The negative anchor position of the icon. [10,20] means the left top of the icon is 10px left of the lat/lng center, and 20px above the lat/lng center. Without iconAnchor values, the icon is centered on the lat/lng center horizontally and vertically. // 'backgroundPosition' => '5px 10px' // Optional. The CSS background-position of the icon images, including 'px'. Default= '0,0'. ]; } return $settings; } );
The following example shows how to use one SVG image for all five cluster image sizes. It also sets five custom ascending image sizes and text sizes, and sets the text color to white:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $settings ) { $clustericonsizes = array( 40, 45, 50, 55, 60 ); // specify custom icon image sizes $clustericontextsizes = array( 12, 14, 16, 18, 20 ); // specify custom icon text sizes for ( $i = 1; $i <= 5; $i ++ ) { $settings['config']['cluster']['styles'][] = [ 'url' => 'https://yourdomain/wp-content/themes/yourtheme/yourimages/clustericon.svg', // custom directory, required. The url becomes the CSS background-image url. 'width' => $clustericonsizes[ $i - 1 ], // required 'height' => $clustericonsizes[ $i - 1 ], // required 'textColor' => '#ffffff', // white, optional 'textSize' => $clustericontextsizes[ $i - 1 ], // optional // 'anchor' => [10,20], // Optional. Anchor position of the label text. By default label text is centered horizontally and vertically in the icon. [10,20] means 10px from the top of the icon (y) and 20px (x) from the left of the icon. Values need to be smaller than width and height of the icon to take effect. // 'iconAnchor' => [10,20], // Optional. The negative anchor position of the icon. [10,20] means the left top of the icon is 10px left of the lat/lng center, and 20px above the lat/lng center. Without iconAnchor values, the icon is centered on the lat/lng center horizontally and vertically. // 'backgroundPosition' => '5px 10px' // Optional. The CSS background-position of the icon images, including 'px'. Default= '0,0'. ]; } return $settings; } );
Method 3: Using cssClass
The third way of customizing cluster icons is by using the cssClass
option of the marker clusterer library. The cluster “icons” in the code will then consist of a div
with the custom class you set, and will contain only the cluster icon text (the number of markers in the cluster):
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { if ( isset( $args['config']['cluster'] ) ) { $args['config']['cluster']['cssClass'] = 'my-cluster-class'; } return $args; } );
You can then style the cluster icons in any way you like. Below is an example of accompanying CSS to style the
.my-cluster-class
div, resulting in a cluster icon looking like the image on the right.
Be aware that the position: absolute;
rule is not optional.
How to use custom CSS?
CSS code can be placed in your (child) theme's style.css file. Alternatively, you can add it manually between
<style>
tags in the<head>
section, in your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More info.my-cluster-class { position: absolute; /* not optional */ width: 36px; height: 36px; border-radius: 50%; background: #ff0000; border: 2px solid #fff; cursor: pointer; color: #fff; font-weight: bold; font-size: 14px; line-height: 32px; text-align: center; }
Exclude the Proximity marker pin from marker clustering
If you are using the map with a Proximity facet, a yellow location marker is displayed on the map when a location is entered. This Proximity marker will be part of the closest marker cluster and will also be counted in the cluster’s displayed number.
To exclude the Proximity marker pin from the clustering behavior, add the following code to your (child) theme’s functions.php:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_footer', function() { ?> <script type="text/javascript"> (function() { document.addEventListener('facetwp-loaded', function() { var markerClusterer = FWP_MAP.mc; var proximitymarker = markerClusterer.getMarkers().find(m => typeof m.post_id === 'undefined'); if (proximitymarker) { markerClusterer.removeMarker(proximitymarker); proximitymarker.setMap(FWP_MAP.map); } }); })(); </script> <?php }, 100 );
It is also possible to disable the Proximity marker pin entirely.
Customize cluster click behavior
If you want to customize the functionality of the map clusters, the following code shows how to access the marker cluster click event.
Two basic examples are shown: the first uses fitBounds()
to zoom into a cluster when it is clicked, which essentially does the same as setting zoomOnClick to true. The second example gets all markers in the clicked cluster, with the getMarkers()
method.
More methods that can be used with the marker clusterer library can be found here.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php add_action( 'wp_footer', function() { ?> <script type="text/javascript"> (function($) { ClusterIcon.prototype.triggerClusterClick = function() { var markerClusterer = this.cluster_.getMarkerClusterer(); // Trigger the clusterclick event google.maps.event.trigger(markerClusterer, 'clusterclick', this.cluster_); // Your code here. Two examples: // Example 1: Zoom in to bounds of cluster. Does the same as when zoomOnClick is set to true this.map_.fitBounds(this.cluster_.getBounds()); // Example 2: Put all markers in the clicked cluster into an object var markers = this.cluster_.getMarkers(); console.log(markers); } })(jQuery); </script> <?php }, 100 );
Overlapping Marker Spiderfier
The Map facet has a feature that helps deal with markers that are close together or overlap because they share (almost) the same latitude / longitude.
The built-in “Overlapping Marker Spiderfier” makes markers fan out (“spiderfy”) into a circle with spokes, on click. Larger numbers (more than 8) fan out into a more space-efficient spiral.
Overlapping Marker Spiderfier plays nice with marker clustering: once you get down to a zoom level where individual markers are shown, these markers then spiderfy.
You may need to adapt the maxZoom parameter on the clusterer though, to ensure that it doesn’t cluster close markers all the way to the level that spiderfying would be more helpful behavior.
Customize Overlapping Marker Spiderfier
The Overlapping Marker Spiderfier library that FacetWP uses, has several useful options that determine how the ‘spider parts’ look and behave.
For example, setting the keepSpiderfied
option to false
overrides the default behavior where the spiderfied markers stay ‘spiderfied’ when you click a marker.
And the circleSpiralSwitchover
option sets the number of markers at which the marker configuration turns into a spiral instead of a circle.
You can also set the leg length, leg thickness and several spiral properties. The code below shows some examples:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { if ( isset( $args['config']['spiderfy'] ) ) { // 'Unspiderfy' when clicking a 'spiderfied' marker $args['config']['spiderfy']['keepSpiderfied'] = false; // (FacetWP's) default: true // Do not 'unspiderfy' when clicking an empty spot on the map $args['config']['spiderfy']['ignoreMapClick'] = true; // default: false // Set the pixel radius within which a marker is considered to be overlapping a clicked marker. $args['config']['spiderfy']['nearbyDistance'] = 30; // default: 20 // Switch from circular to spiral above 11 markers instead of above 8 $args['config']['spiderfy']['circleSpiralSwitchover'] = 11; // default: 8 // Set spider leg length when circular $args['config']['spiderfy']['circleFootSeparation'] = 40; // default: 23 // Set spider leg length and other settings when spiral $args['config']['spiderfy']['spiralFootSeparation'] = 40; // default: 26 $args['config']['spiderfy']['spiralLengthStart'] = 15; // default: 11 $args['config']['spiderfy']['spiralLengthFactor'] = 6; // default: 4 // Set spider leg thickness $args['config']['spiderfy']['legWeight'] = 3; // default: 1.5 } return $args; } );
Note that the FacetWP Map facet sets the following options to true
by default, overriding the defaults mentioned in the Overlapping Marker Spiderfier library’s documentation:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
// FacetWP Map facet Spiderfier defaults: 'spiderfy' => [ 'markersWontMove' => true, 'markersWontHide' => true, 'basicFormatEvents' => true, 'keepSpiderfied' => true ]
Customize spider leg colors
The following example shows how to set the color and highlight color of the spider legs. The colors need to be set for each map type separately. This examples only sets the colors for the ROADMAP
map type. See the defaults and map type options here.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_footer', function () { ?> <script> document.addEventListener('facetwp-loaded', function() { if ('undefined' !== typeof FWP_MAP) { var mti = google.maps.MapTypeId; FWP_MAP.oms.legColors.usual[mti.ROADMAP] = '#cc52ab'; // Normal leg color. Default: '#444' FWP_MAP.oms.legColors.highlighted[mti.ROADMAP] = '#6233ca'; // Highlight leg color. Default '#f00' } }); </script> <?php }, 100 );
Map marker hooks and functions
FacetWP provides several JavaScript hooks and functions to perform actions when markers are interacted with, or to perform actions on markers, for example when markers themselves, post (titles) in the result listing, or infoWindows close buttons are clicked.
Example 1: Add a class to the post which has its marker clicked
The following example adds the CSS class is-active
to the active post (the post which has its corresponding marker clicked), which could be used to style it differently than the other posts:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php add_action( 'wp_footer', function() { ?> <script> (function($) { if ('object' !== typeof FWP) { return; } FWP.hooks.addAction('facetwp_map/marker/click', function(marker) { /** * The post ID associated with the active marker */ var post_id = marker.post_id; /** * The following code adds the CSS class "is-active" to the active post, * assuming each result's HTML is structured similarly to: * <div class="post-item" data-id="123">Hello World!</div> */ $('.post-item').removeClass('is-active'); $('.post-item[data-id="' + post_id + '"]').addClass('is-active'); }); })(fUtil); </script> <?php }, 100 );
The above example assumes your posts (or post titles) have a class post-item
and a data-id
attribute containing the post ID. One way to accomplish this is by using the Listing Builder’s Dev mode and adding the following code to the “Display Code” field:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php while ( have_posts() ): the_post(); ?> <h3><a href="<?php the_permalink(); ?>" class="post-item" data-id="<?php the_id(); ?>"><?php the_title(); ?></a></h3> <?php endwhile; ?>
Example 2: Open a marker’s infoWindow on mouse hover
In addition to the click
event, FacetWP also supports mouseover
and mouseout
events.
This example opens a marker’s infoWindow on mouse hover:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_footer', function() { ?> <script> (function($) { if ('object' !== typeof FWP) { return; } FWP.hooks.addAction('facetwp_map/marker/mouseover', function(marker) { FWP_MAP.infoWindow.setContent(marker.content); FWP_MAP.infoWindow.open(FWP_MAP.map, marker); }); })(fUtil); </script> <?php }, 100 );
Example 3: Trigger a marker click and infoWindow on post click
The get_post_markers()
function can be used to get markers by post ID and perform actions on them.
The following example triggers a marker click when you click on a post in the results list. It sets the map center to the marker’s position, opens its infoWindow and sets a custom zoom level.
Make sure that the zoom level is higher than the marker clusterer’s bottom limit, otherwise the separate markers will not be shown.
The click event to use is spider_click
because of the built-in Overlapping Marker Spiderfier. If you don’t have any markers close together, you can also use the click
event.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php add_action( 'wp_footer', function () { ?> <script> (function($) { $(document).on('click', '.post-item', function(e) { e.preventDefault(); // Necessary if '.post-item' is an <a> tag and you don't want it to open the post page itself. var postid = $(this).attr('data-id'); var marker = FWP_MAP.get_post_markers(postid); $.each( marker, function( key, value ) { FWP_MAP.map.setCenter({ lat: value.position.lat(), lng: value.position.lng() }); FWP_MAP.is_zooming = true; // Needed if you are using the "Enable map filtering" button FWP_MAP.map.setZoom(17); // Set a zoom level between 1 and 20. Make sure it is higher than the marker clusterer's bottom limit. // google.maps.event.trigger(value, 'click'); // If you don't have spiderfied markers google.maps.event.trigger(value, 'spider_click'); // If you have spiderfied markers. Will also work if you don't have spiderfied markers. }); }); })(jQuery); </script> <?php }, 100 );
The above example assumes your posts items (or post titles) have a class post-item
and a data-id
attribute containing the post ID. See the second code block in example 1 above to see how to accomplish that with the Listing Builder’s Dev mode.
Example 4: Change the marker icon when an infoWindow is open
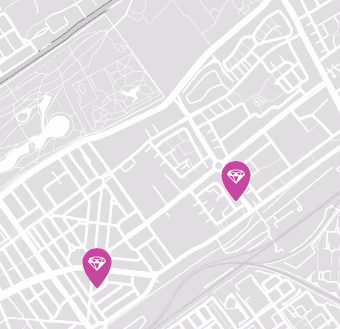
This code example changes the (custom) marker icon when an infoWindow is opened and switches it back when it is closed. This can be useful to indicate an ‘active’ marker with a different design or color.
First we define the ‘default’ and ‘active’ icons, using the properties of the google.maps.Icon interface. The specified ‘default’ icon and its properties should of course be the same as the custom icon you have specified with one of the above methods.
We use the facetwp_map/marker/click
hook to detect a click on a marker and store its post_id
to get its object. In that object we then set that marker’s icon to the ‘active’ version, with the setIcon() method.
We use Google Map API’s closeclick event to detect the closing of the infoWindow. When that happens, or when the map is clicked anywhere (causing all infoWindows to close), or when another marker is clicked, we use FacetWP’s get_post_markers
function and pass it the stored active marker’s post_id
to get the that marker’s object so we can switch its icon back to the original one:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_footer', function() { ?> <script> (function($) { if ('object' !== typeof FWP) { return; } // Change URL to the default marker icon let icon_default = { url: '/wp-content/themes/my-theme/assets/icons/facetwp-marker.svg', scaledSize: { width: 40, height: 40 } }; // Change URL to the alternative, 'active' icon let icon_active = { url: '/wp-content/themes/my_theme/assets/icons/facetwp-marker-active.svg', scaledSize: { width: 40, height: 40 } }; $(function() { FWP.hooks.addAction('facetwp_map/marker/click', function(marker) { // Check if another marker is already active. If so set to default icon if (window.marker_post_id) { let post_id = window.marker_post_id; let marker = FWP_MAP.get_post_markers(post_id)[0]; marker.setIcon(icon_default); } // Set the clicked marker to have the 'active' icon window.marker_post_id = marker.post_id; marker.setIcon(icon_active); }); // When an infoWindow is closed revert its marker to the default icon google.maps.event.addListener(FWP_MAP.infoWindow, 'closeclick', function() { let post_id = window.marker_post_id; let marker = FWP_MAP.get_post_markers(post_id)[0]; marker.setIcon(icon_default); }); // When the map is clicked anywhere, revert the active marker to the default icon google.maps.event.addListener(FWP_MAP.map, 'click', function(event) { if (window.marker_post_id) { let post_id = window.marker_post_id; let marker = FWP_MAP.get_post_markers(post_id)[0]; marker.setIcon(icon_default); } }); }); })(jQuery); </script> <?php }, 100 );
How to add a Map facet to single posts or pages
We often get the question if it is possible to use the Map add-on to show a map on a single page, post, or custom post type post. A use case would be to show the location of a store, hotel, restaurant, or event.
This is possible, and actually not very complicated. Just follow the steps described in this tutorial.