facetwp_builder_item_value
Overview
This filter lets you modify the output value of a Listing Builder item.
Parameters
- $value | mixed | The item value
- $item | array | An associative array of item data:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
$item = [ 'source' => 'cf/photo_url', 'settings' => [ 'prefix' => '', 'suffix' => '', 'is_hidden' => false, 'name' => 'photo_url', 'css_class' => '', // other settings based on the item type ] ];
Within this hook, you also have access to the current post, for example:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
global $post; $post_id = $post->ID; $post_id = get_the_ID(); $post_url = get_the_permalink();
Another feature that makes this hook very powerful is that you can use built-in and custom dynamic tags within it. The built-in tags get post properties like the URL or title. Custom dynamic tags can get content from other (hidden) builder items, which gives you access to content from custom fields:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
// built-in dynamic tags {{ post:id }} // Returns the post’s id {{ post:name }} // Returns the post’s name (slug) {{ post:type }} // Returns the post’s type {{ post:title }} // Returns the post’s title {{ post:url }} // Returns the post’s url {{ post:image }} // Returns the post’s featured image (in "full”" size). // custom dynamic tags {{ my-builder-item-name }}
Check out the example below to see how to implement these dynamic tags.
Examples
Below are a few examples that show how versatile this hook makes the Listing Builder, and how creative you can get.
Limit a builder item to a number of characters
This example takes a Post Excerpt builder item, limits its output to 120 characters, and appends an ellipsis between parentheses (…) to the end of the string:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_builder_item_value', function( $value, $item ) { if ( 'post_excerpt' == $item['source'] ) { $value = substr( $value, 0, 120 ).' (…)'; } return $value; }, 10, 2 );
Be aware that the above example can cause problems if the excerpt string contains HTML. The substr
function trims to the set number of characters. It does not recognize HTML and could leave broken HTML tags.
If you have HTML in your excerpts, you could also use wp_trim_words
to trim the item to a specific number of words:
Limit a builder item to a number of words
This example selects a builder item by name and limits its output to 8 words (not characters) with the wp_trim_words function. It uses the third parameter of this function to append an ellipsis between parentheses (…) after the last word:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_builder_item_value', function( $value, $item ) { if ( 'my-excerpt' == $item['settings']['name'] ) { $length = 8; // set the number of words $more = ' (…)'; // append an ellipsis between parentheses (…) after the last word $value = wp_trim_words( $value, $length, $more ); } return $value; }, 10, 2 );
Add a read more link to a Post Excerpt builder item
This example takes a Post Excerpt builder item, trims it to 8 words, then adds an ellipsis between parentheses (…) and a “read more” link to the post URL:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_builder_item_value', function( $value, $item ) { if ( 'post_excerpt' == $item['source'] ) { $length = 8; // set the number of words $more = '<a href="' . get_permalink() . '" class="readmorelink"> (…) read more</a>'; // Append an ellipsis with a read more link to the post URL $value = wp_trim_words( $value, $length, $more ); } return $value; }, 10, 2 );
Change the date format from a custom field
The following example takes the value from a builder item made from a custom field with a date/time string, and changes the format:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_builder_item_value', function( $value, $item ) { if ( 'date-field' == $item['settings']['name'] ) { $value = date_create( $value ); $value = date_format( $value, "d-m-Y" ); } return $value; }, 10, 2 );
Using array fields – output a comma-separated string with line breaks instead
If the source of the builder item is a custom field that outputs an array (for example an ACF Checkboxes or Taxonomy field), FacetWP will automatically output the array values as a comma-separated string. This array-to-string conversion takes place after the facetwp_builder_item_value
hook has already run. So if you want to override this comma-separated string output, you’ll have to do the array-to-string conversion within the hook itself.
The following example shows how to convert the array output from the my-item-name
builder item to a string with <br/>
tags, making each item display on its own line. The delimiter can be changed to any character or HTML:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_builder_item_value', function( $value, $item ) { if ( $item['settings']['name'] == 'my-item-name' ) { // Change "my-item-name" to the name of your builder item. if ( is_array( $value ) && ! empty( $value ) ) { return implode( '<br />', $value ); // '<br />' is the delimiter. This can be changed to any character or HTML. } else { return ''; } } return $value; }, 1000, 2 ); // Use priority 1000 for ACF array fields.
Note that for this to work on all types of ACF array fields, the hook needs to run at priority 1000
, because FacetWP’s own processing of ACF custom field values also uses this hook, at priority 999
.
Add a link to specific builder items
Some builder item types have their own “Link” setting to link them to the post URL (or a custom URL), but others, like the ones made from Advanced Custom Fields or Pods custom fields, do not. This hook can be used to add a link to those kinds of items.
The following example adds a link to the builder item with the name my-item-name
:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php // replace 'my-item-name' with the name of your builder item add_filter( 'facetwp_builder_item_value', function( $value, $item ) { if($item['settings']['name'] == 'my-item-name') { return '<a href="' . get_permalink() . '">' . $value . '</a>'; } return $value; }, 10, 2 );
Within the hook you can also access other post properties. You could for example retrieve the post ID with get_the_ID()
in order to link each item to an external website with the post ID as part of the link:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php // replace 'my-item-name' with the name of your builder item add_filter( 'facetwp_builder_item_value', function( $value, $item ) { if ( $item['settings']['name'] == 'my-item-name' ) { return '<a href="https://my-other-website.com/products/' . get_the_ID() . '" target="_blank" rel="nofollow noopener">' . $value . '</a>'; } return $value; }, 10, 2 );
Add a post author link to a Post Author builder item
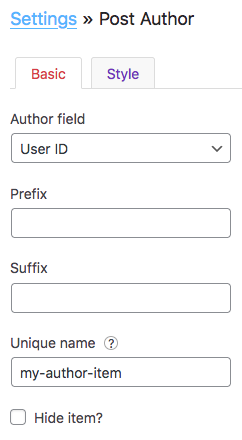
The following example shows how to add a post author link to a Post Author builder item.
First, set your Post Author Listing Builder item’s ‘Author field’ setting to ‘User ID’, as shown in the image on the right. Also give the item a unique name.
Then add the following snippet to your (child) theme’s functions.php, and replace my-author-item
with the ‘Unique name’ of your Post Author builder item:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
// Set your Post Author Listing Builder item's 'Author field' setting to 'User ID' // Replace 'my-item-name' with the 'Unique name' of your Post Author builder item add_filter( 'facetwp_builder_item_value', function( $value, $item ) { if( $item['settings']['name'] == 'my-author-item' ) { $author_id = $value; $author_link = get_author_posts_url( $author_id ); $author_name = get_the_author_meta( 'display_name', $author_id ); return '<a href="' . $author_link . '">'. $author_name . '</a>'; } return $value; }, 10, 2 );
Add a WooCommerce “Add to cart” button to product listing items
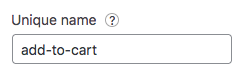
add-to-cart
.To add a WooCommerce “Add to cart” button to each product in a product listing, add a Listing Builder item (any type will work, e.g. a Button or HTML item), and set its ‘Unique name’ to add-to-cart
.
Then, add the following snippet to your (child) theme’s functions.php:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_builder_item_value', function( $value, $item ) { if ( 'add-to-cart' == $item['settings']['name'] ) { global $post; $value = do_shortcode( '[add_to_cart id="' . $post->ID . '"]' ); } return $value; }, 10, 2 );
Use dynamic tags
The following example demonstrates how to use dynamic tags within this hook. In this case we used two types of tags: a built-in dynamic tag, {{ post:url }}
, which generates the post URL in the href
attribute. And a custom dynamic tag, {{ my-item-price }}
, made from a separate (hidden) builder item by that name, which retrieves the price from a custom field. The resulting output is a link to the post URL with the link text containing the value of the my-item-name
builder item (which could be the post title), plus the price.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php // replace 'my-item-name' and 'my-item-price' with the names of your builder items add_filter( 'facetwp_builder_item_value', function( $value, $item ) { if($item['settings']['name'] == 'my-item-name') { return '<a href="{{ post:url }}">' . $value . ' - $ {{ my-item-price }}</a>'; } return $value; }, 10, 2 );
Output a simple HTML5 audio player from a file upload field
This creative example outputs a simple HTML5 audio / mp3 player using a builder item made from an ACF file upload field. The builder item needs to have the ‘Unique name’ mp3-preview
.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php add_filter( 'facetwp_builder_item_value', function( $value, $item ) { if ( 'mp3-preview' == $item['settings']['name'] ) { if ( is_array( $value ) && ! empty( $value['url'] ) ) { $src = $value['url']; $value = '<audio controls>'; $value .= '<source src="' . $src . '" type="audio/mpeg">'; $value .= 'Your browser does not support the audio element.'; $value .= '</audio>'; } } return $value; }, 10, 2 );