facetwp_facet_render_args
Overview
This filter lets you modify the data passed to a facet’s render() method. This is useful if you need to alter the selected values, or translate facet choices.
Parameters
- $args | array | An associative array of facet render arguments (see below)
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
$args = [ 'facet' => [ 'label' => 'Model Year', 'name' => 'model_year', 'type' => 'checkboxes', ... ], 'where_clause' => 'AND post_id IN (18670,18671,18672,18673,18674)', 'selected_values' => [ '2014', '2015', '2016' ] ];
Some facet types also have $args['values']
, depending on whether the facet type has a load_values()
method.
Usage examples
Modify selected facet values
Modify the selected values for a specific facet (e.g. model_year
):
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facet_render_args', function( $args ) { if ( 'model_year' == $args['facet']['name'] ) { $args['selected_values'] = [ '2015' ]; } return $args; });
Translate facet choices and facet settings
This hook can also be used to dynamically change facet choices and/or facet settings into __()
translatable strings. Which then can be translated with a string translation plugin.
Before you go this route, note that you can also use the facetwp_facet_display_value hook to translate facet choices.
And, see this section on the Multilingual add-on page for more info about the recommended way to translate facet choices, which is to translate the facet’s data source itself (the custom field or taxonomy terms).
Translate facet choices
The following example changes specific facet choices within the facet named country
to __()
translatable strings:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facet_render_args', function( $args ) { if ( 'country' == $args['facet']['name'] ) { // Change 'country' to the name of your facet $translations = [ 'Spain' => __( 'Spain', 'fwp-front' ), 'United States' => __( 'United States', 'fwp-front' ), 'France' => __( 'France', 'fwp-front' ) ]; if ( ! empty( $args['values'] ) ) { foreach ( $args['values'] as $key => $val ) { $display_value = $val['facet_display_value']; if ( isset( $translations[ $display_value ] ) ) { $args['values'][ $key ]['facet_display_value'] = $translations[ $display_value ]; } } } } return $args; });
Alternatively, change all facet choices in the country
into __()
translatable strings:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facet_render_args', function( $args ) { if ( 'country' == $args['facet']['name'] ) { // Change 'country' to the name of your facet if ( ! empty( $args['values'] ) ) { foreach ( $args['values'] as $key => $val ) { $display_value = $val['facet_display_value']; $args['values'][ $key ]['facet_display_value'] = __( $display_value, 'fwp-front' ); } } } return $args; });
These dynamically added strings can now be translated with a string translation plugin.
Translate Pager facet labels
The following example changes all labels that Pager facets use, into __()
translatable strings:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facet_render_args', function( $args ) { if ( 'pager'== $args['facet']['type'] ) { $args['facet']['prev_label'] = __('« Prev', 'fwp-front' ); $args['facet']['next_label'] = __('Next »', 'fwp-front' ); $args['facet']['dots_label'] = __('…', 'fwp-front' ); $args['facet']['count_text_plural'] = __('[lower] - [upper] of [total] results', 'fwp-front' ); $args['facet']['count_text_singular'] = __('1 result', 'fwp-front' ); $args['facet']['count_text_none'] = __('No results', 'fwp-front' ); $args['facet']['load_more_text'] = __('Load more', 'fwp-front' ); $args['facet']['loading_text'] = __('Loading...', 'fwp-front' ); $args['facet']['default_label'] = __('Per page', 'fwp-front' ); $args['facet']['per_page_options'] = __('10, 25, 50, 100, Show all', 'fwp-front' ); } return $args; });
These dynamically added strings can now be translated with a string translation plugin.
Note that these Pager facet labels can also be translated with the facetwp_i18n hook. And the Pager facet links’ HTML can be customized with the facetwp_facet_pager_link hook.
How to translate dynamically added strings
With the above snippets in your (child) theme’s functions.php, these dynamically added __()
strings for facet choices and facet setting labels can now be translated with a string translation plugin.
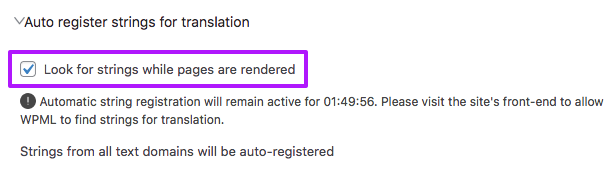
For WPML, use WPML String translation. To let WPML auto-detect these new strings, go to: WPML > String Translation > Auto register strings for translation. Enable the “Look for strings while pages are rendered”, and (within the time frame the setting is active), visit the page where the facets are. The new facet choice strings will then be detected and added to the fwp-front
domain. Disable the setting again when all strings have been detected.
Another useful plugin for string translations is Loco Translate. If needed, you can use it together with a tool like PoEdit to handle or create the language pot/po/mo files.
Translatable strings can also be translated with a gettext
filter, as shown in the following example:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'gettext', function( $translated_text, $text, $domain ) { if ( 'fwp-front' == $domain ) { if ( 'Spain' == $text ) { $translated_text = 'Spanje'; } if ( 'United States' == $text ) { $translated_text = 'Verenigde Staen'; } if ( 'France' == $text ) { $translated_text = 'Frankrijk'; } } return $translated_text; }, 10, 3 );
Customize a facet setting
The following example shows how to programmatically customize the “Default radius” setting in a Proximity facet, only on a specific page.
Make sure to change the page ID on line 2 (or use another conditional tag). And change my_proximity_facet
on line 3 to the name of your Proximity facet:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facet_render_args', function( $args ) { if ( is_page( 4956 ) ) { // Change to your page ID or use another condition if ( 'my_proximity_facet' == $args['facet']['name'] ) { // Change 'my_proximity_facet' to the name of your Proximity facet $args['facet']['radius_default'] = 300; // Set the default radius } } return $args; });
Note that if the Proximity facet’s “Radius UI” setting is set to “Slider”, the custom default radius set in line 4 must be within the range of the “Range (min)” and “Range (max)” setting.
If the Proximity facets “Radius UI” setting is set to “Dropdown”, the custom radius will be appended at the bottom of the list of choices if it is not one of the defined choices in the “Radius options” setting.