facetwp_builder_dynamic_tag_value
Overview
This filter lets you create your own custom dynamic tags, or modify the value of built-in or custom dynamic tags, to be used in the Listing Builder.
This hook calculates values on-the-fly. Unlike the facetwp_builder_dynamic_tags hook, if a post doesn’t use a given tag, then its value won’t be calculated, which is more efficient.
Parameters
- $tag_value | string | The tag value
- $tag_name | string | The tag name
- $params | array | An associative array of extra data:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
$params['layout'] // the raw layout data for this template $params['post'] // the current post
Create new custom dynamic tags
Below are two examples of how to create your own custom dynamic tag. You can make up the tag name yourself, {{ myamazingtag }}
is entirely valid.
Create a dynamic tag to output the value of a custom field
The following example creates a custom dynamic tag to output an ACF custom field named first_name
:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_builder_dynamic_tag_value', function( $tag_value, $tag_name, $params ) { if ( 'acf:firstname' == $tag_name ) { $tag_value = get_field( 'first_name', $params['post']->ID ); } return $tag_value; }, 10, 3 );
Then within the Listing Builder, you can use {{ acf:firstname }}
to output the first name.
Create a dynamic tag that outputs terms as classes
The following example creates a custom dynamic tag {{ resource_type }}
that outputs the terms of the resource-type
taxonomy for the current post. The terms are output as space-separated slugs, to be used as classes in an HTML builder element in a Listing Builder listing.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_builder_dynamic_tag_value', function( $tag_value, $tag_name, $params ) { if ( 'resource_type' == $tag_name ) { $terms = wp_get_post_terms( $params['post']->ID, 'resource-type', array( 'fields' => 'slugs' ) ); $tag_value = implode( ' ', $terms ); } return $tag_value; }, 10, 3 );
This new dynamic tag can now be used in an HTML element. The following HTML adds a <div>
with the post type and all current terms from the resource-type
taxonomy as classes. Note that {{ post:type }}
is a built-in dynamic tag.
<div class="{{ post:type }} {{ resource_type }}"></div>
Modify existing dynamic tags
Below are a few examples of how to modify the output of existing tags and how to apply them:
Change the image size for the built-in ‘post:image’ tag
This example changes the image size used by the built-in {{ post:image }}
dynamic tag from the default 'full'
, to 'medium'
size. You can use any default or custom WordPress image size.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_builder_dynamic_tag_value', function( $tag_value, $tag_name, $params ) { if ( 'post:image' == $tag_name ) { $tag_value = get_the_post_thumbnail_url( $params['post']->ID, 'medium' ); // Default size is 'full' } return $tag_value; }, 10, 3 );
Change the image size for the built-in ‘post:image’ tag with fallback image
The example does the same as the previous example, but with a fallback image. Change http://example.com/image.jpg
to an image to use when no image URL is found:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_builder_dynamic_tag_value', function( $tag_value, $tag_name, $params ) { if ( 'post:image' == $tag_name ) { $tag_value = ( $image = wp_get_attachment_image_url( get_post_thumbnail_id(), 'medium' ) ) ? esc_url( $image ) : 'http://example.com/image.jpg'; // Default size is 'full' } return $tag_value; }, 10, 3 );
Modify the post title with the built-in ‘post:title’ tag
This example uses the built-in {{ post:title }}
dynamic tag, and modifies it to prepend the post title with “Sale: ” if a product has the term “sale” selected in the Product Category (product_cat
) taxonomy:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_builder_dynamic_tag_value', function( $tag_value, $tag_name, $params ) { if ( 'post:title' == $tag_name && has_term('sale', 'product_cat')) { $tag_value = 'Sale: '. get_the_title( $params['post']->ID); } return $tag_value; }, 10, 3 );
Modify a custom dynamic tag made within the Listing Builder
This example shows how you can modify the value of a dynamic tag that was made with a hidden builder item within the Listing Builder itself.
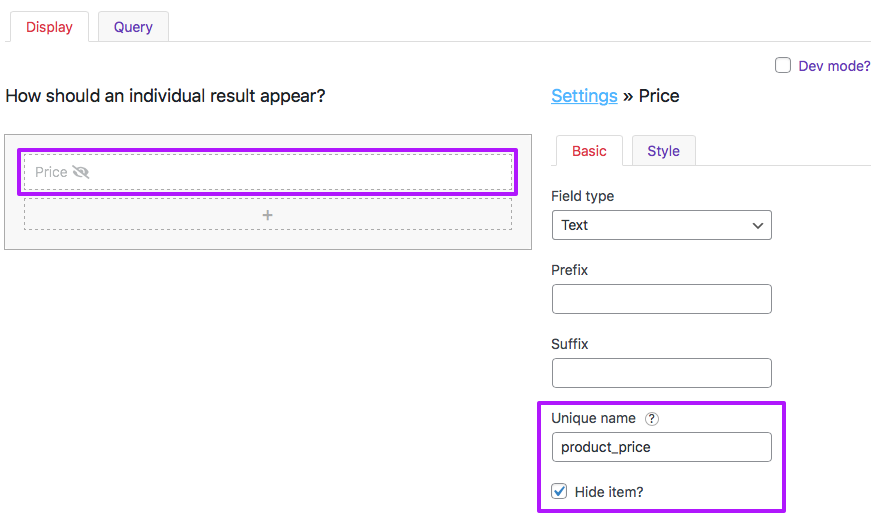
The created dynamic tag {{ product_price }}
pulls the price from the Price
custom field. The example below shows how to modify the output of this tag so that the price is prepended with a €
currency sign:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_builder_dynamic_tag_value', function( $tag_value, $tag_name, $params ) { if ( 'product_price' == $tag_name) { $tag_value = '€ '. $tag_value; } return $tag_value; }, 10, 3 );
Using a custom dynamic tag in the Listing Builder
An example of how to use two of the above examples in the Listing Builder would be to create an HTML builder item with the modified post title in a <h3>
heading, and a div
that uses the featured image url as CSS background image:
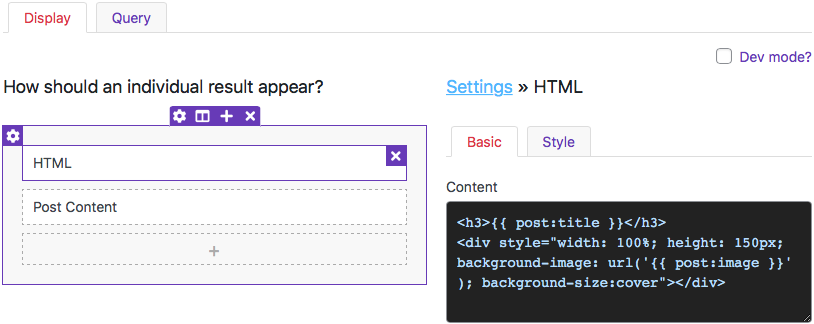