User Post Type
Create filterable user listings
This plugin lets you connect users to a post type, so that you can create user listings, and filter users like any other post type.
How it works
The plugin adds a new hidden upt_user
post type, and adds a post for each user.
Setup
Download and install the add-on from your account. Because User Post Type is a premium add-on, the download link is on a different page than other add-ons. In your account, click the “View Details and Downloads” link beside your purchase in the “Purchase history” table. Scroll down, and the User Post Type download link should be right below the one for FacetWP itself.
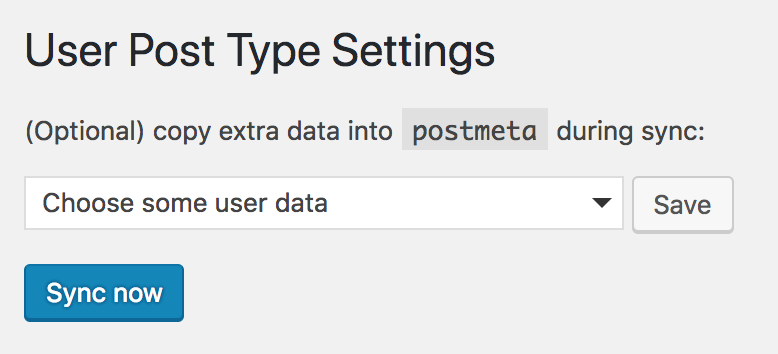
After activating the plugin, browse to Settings > User Post Type
and click the “Sync now” button.
As seen in the image on the right, you can also copy extra data into the postmeta table. This is not required to display fields – it’s only useful for meta_query.
Here’s a list of dropdown choices and corresponding meta_query
keys:
Option | Meta Key |
---|---|
User ID | ID |
User Login | user_login |
User Email | user_email |
User URL | user_url |
Registration Date | user_registered |
User Status | user_status |
Display Name | display_name |
Roles | roles |
Usermeta field | meta-zipcode (keys are prefixed with meta- ) |
BuddyPress field | bp-123 (field IDs are prefixed with bp- ) |
Displaying users
To display a list of users, use WP_Query
(not WP_User_Query
) like so:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
$args = [ 'post_type' => 'upt_user', 'post_status' => 'publish', 'posts_per_page' => 10, ]; $query = new WP_Query( $args );
Displaying a subset of users
To display only administrators, select “Roles” in the settings page dropdown, then Sync. Afterwards, use the field within meta_query
:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
$args = [ 'post_type' => 'upt_user', 'post_status' => 'publish', 'orderby' => [ 'title' => 'ASC' ], 'posts_per_page' => 30, 'meta_query' => [ [ 'key' => 'roles', 'value' => 'administrator', 'compare' => '=', ] ] ]; $query = new WP_Query( $args );
The same with multiple roles, in this example administrators and editors:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
$args = [ 'post_type' => 'upt_user', 'post_status' => 'publish', 'orderby' => [ 'title' => 'ASC' ], 'posts_per_page' => 30, 'meta_query' => [ [ 'key' => 'roles', 'value' => [ 'administrator', 'editor' ], 'compare' => 'IN', ] ] ]; $query = new WP_Query( $args );
Displaying user fields
UPT supports WP’s get_user_meta() function. Just replace $user_id
with UPT()->get_user_id()
:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
echo get_user_meta( UPT()->get_user_id(), 'your_field_name', true );
Outside of The Loop, pass in the post ID like so:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
echo get_user_meta( UPT()->get_user_id( THE_POST_ID ), 'your_field_name', true );
To get a user’s photo, you can use WP’s get_avatar() function, assuming that the image is stored in WP’s default “Profile Picture” field:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
echo get_avatar( UPT()->get_user_id( THE_POST_ID ), 150 );
Adding facets
When adding a new facet via Settings > FacetWP
, you’ll see a new “User Fields” section in the Data source dropdown.
Finding a user’s associated post
Use the following if you have a $user_id
and want to find the $post_id
of the associated post:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
$post_id = (int) get_user_meta( $user_id, UPT()->meta_key, true );
Prevent a specific user from being synced
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'upt_sync_skip_user', function( $bool, $user_id ) { if ( 1 === $user_id ) { return true; } return $bool; }, 10, 2 );
Prevent users with specific roles from being synced
To prevent users with a specific role from being synced, you can use the following snippet. Change the roles to be skipped in the array in line 3.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'upt_sync_skip_user', function( $bool, $user_id ) { $roles = (array) get_user_by( 'ID', $user_id )->roles; $roles_to_skip = [ 'dormant-student', 'dormant-teacher' ]; // Skip these roles foreach ( $roles_to_skip as $role ) { if ( in_array( $role, $roles ) ) { return true; // Do not sync this user } } return $bool; }, 10, 2 );
This also works for custom user roles made with a plugin, like for example User Role Editor.
You can also do the opposite:
Only sync users with a specific role
To sync only users with a specific role, you can use the following snippet. Change the roles to be synced in the array in line 3.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'upt_sync_skip_user', function( $bool, $user_id ) { $roles = (array) get_user_by( 'ID', $user_id )->roles; $roles_to_preserve = [ 'student','teacher' ]; // Sync only these roles $intersect = array_intersect( $roles, $roles_to_preserve ); if ( empty( $intersect ) ) { return true; // Do not sync this user } return $bool; }, 10, 2 );
Perform post-sync actions
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'upt_sync_post', function( $post_id, $user_id ) { // do something after a user is synced }, 10, 2 );
Force only certain users to be indexed
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'upt_sync_where', function( $where ) { $where = ' AND u.ID IN (1, 2, 3, 4, 5)'; return $where; });
Force a user sync on a schedule
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'mysite_sync_users', 'mysite_sync_users_callback' ); function mysite_sync_users_callback() { UPT()->sync->run_sync(); } if ( ! wp_next_scheduled( 'mysite_sync_users' ) ) { wp_schedule_single_event( time() + 28800, 'mysite_sync_users' ); // 28800 seconds = every 8 hours }
Force a single user sync
To manually sync a single user, use this in your code or hook:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
UPT()->sync->run_sync( $user_id );
Note that users are already synced automatically when their profile is updated from the standard wp-admin user profile editor.
Updating the profile triggers WordPress’ profile_update action hook that the User Post Type add-on uses to trigger the sync. Manual syncing would only be needed if updating a user’s profile is done in a way that does not trigger this hook.
Customize register_post_type arguments for the upt_user post type
You can use the upt_post_type_args
hook to customize any arguments of the register_post_type function, when the upt_user
post type is registered.
Two examples:
Force the admin menu to appear
To force the “UPT Users” admin menu to appear:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'upt_post_type_args', function( $args ) { $args['show_in_menu'] = true; return $args; });
Prevent single upt_user posts from being visible
To prevent the single posts from the upt_user
post type from being accessed on the front-end (via for example https://yourdomain.com/upt_user/user-1/
):
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'upt_post_type_args', function( $args ) { $args['publicly_queryable'] = false; return $args; });
Changelog
0.7.8
- Fixed PHP 8.2 deprecation notices
- Fixed potential name conflict when re-indexing
0.7.7
- Fixed issue preventing value of "0" from getting indexed (props Jenny)
0.7.6
- Important Go into `Settings > User Post Type` and hit the "Sync" button
- Fixed issue causing a new meta row to get added on each user save
0.7.5
- Fixed sync issues (prevents needing to manually re-sync in many cases), props Jenny
0.7.4
- New added `upt_sync_where` hook to customize the SQL WHERE clause
0.7.3
- New added multisite support (only sync users of the current site)