facetwp_facet_sort_options
Overview
This hook lets you customize the Sort facet’s dropdown options and sort logic.
Parameters
- $options | array | Options array (see below)
- $params | array | Associative array of extra input variables (see below)
The $options
array contains an array of settings for each sort option that is set in the Sort facet’s settings. This example has two sort options:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
$options = [ 'title_asc' => [ 'label' => 'Title (a-z)', 'query_args' => [ 'orderby' => [ 'title' => 'ASC' ] ] ], 'title_desc' => [ 'label' => 'Title (z-a)', 'query_args' => [ 'orderby' => [ 'title' => 'DESC' ] ] ], //... ];
The $params
array provides the Sort facet’s settings, and the listing template’s name:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
$params = [ 'facet' => [ 'name' => 'my_sort_facet_name', 'label' => 'My sort facet label', 'type' => 'sort', 'default_label' => 'Sort by', 'sort_options' => [ [ 'label' => 'Title (a-z)', 'name' => 'title_a_z', 'orderby' => [ 'key' => 'title', 'order' => 'ASC', 'type' => 'CHAR', ], ], [ 'label' => 'Title (z-a)', 'name' => 'title_z_a', 'orderby' => [ 'key' => 'title', 'order' => 'DESC', 'type' => 'CHAR', ] ], //... ], 'operator' => 'or', 'selected_values' => [ 'the_selected_sort_value' ] ], 'template_name' => 'my_template_name' ];
Usage
A few examples of how to use this hook:
Remove a sort option
The following code removes the sort option with the name title_z_a
:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facet_sort_options', function( $options, $params ) { unset( $options['title_z_a'] ); return $options; }, 10, 2 );
Remove a sort option conditionally
The following code removes the sort option with the name date
, if the template name is cars
:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facet_sort_options', function( $options, $params ) { if ( 'cars' == $params['template_name'] ) { unset( $options['date'] ); } return $options; }, 10, 2 );
Or, alternatively, use the Sort facet’s name as condition:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facet_sort_options', function( $options, $params ) { if ( 'my_sort_facet' == $params['facet']['name'] ) { unset( $options['date'] ); } return $options; }, 10, 2 );
Add a sort option conditionally
The following code adds a sort option if the template name is my_listing
. You can use any orderby parameter accepted by WP_Query. In this example, we add an option to sort by post type:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facet_sort_options', function( $options, $params ) { if ( 'my_listing' == $params['template_name'] ) { $options['post_type'] = [ 'label' => 'Post type', 'query_args' => [ 'orderby' => [ 'type' => 'ASC' ] ] ]; } return $options; }, 10, 2 );
Add an option to sort by multiple (custom) fields
The following example adds a “Price (Highest)” sort option. As you can see in line 6, the meta_query
has a named key: sort_0
. This name can be anything you want. This key name is then used in the orderby
clause on line 11.
The query will first sort by the custom field _price
, from high to low. This custom field is a numeric field containing decimals. After that it sorts by the secondary, backup sorting method title
. This means that items with the same price will be sorted by post title:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facet_sort_options', function( $options, $params ) { $options['price_desc'] = [ 'label' => 'Price (Highest)', 'query_args' => [ 'meta_query' => [ 'sort_0' => [ 'key' => '_price', 'type' => 'DECIMAL(16,4)', ] ], 'orderby'=> [ 'sort_0' => 'DESC', 'title' => 'ASC' ] ] ]; return $options; }, 10, 2 );
This above added sort option is equivalent to adding it like this in the Sort facet’s settings:
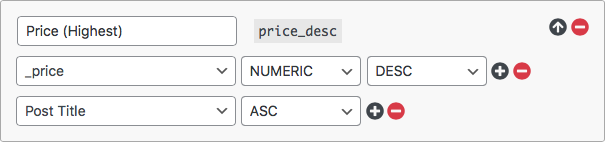
Add an option to sort by a custom field and include posts without that field
If you have set up a sort option that sorts by a custom field, you will notice that posts that do not have that custom field disappear when you use that sort option.
The following snippet shows how to prevent this. You can use this to customize/override the query for an existing sort option, or to create a new sort option.
With this snippet the query first sorts by the custom field, then by post title. Posts without the custom field will be sorted by post title and added after the ones with the custom field:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
// Replace 'my_custom_field' with the name of your custom field. // Replace 'my_custom_field_sort' with an existing sort option's technical name. Or use a non-existing name to create a new sort option. // Replace 'My custom field sort label' with your sort option label. // Use 'meta_value' in line 24 if the custom field does not contain numeric values. add_filter('facetwp_facet_sort_options', function ($options, $params) { $options['my_custom_field_sort'] = [ 'label' => 'My custom field sort label', 'query_args' => [ 'meta_query' => [ 'relation' => 'OR', [ 'key' => 'my_custom_field', 'compare' => 'NOT EXISTS' ], [ 'key' => 'my_custom_field', 'compare' => 'EXISTS' ] ], 'orderby' => [ 'meta_value_num' => 'DESC', // Sort by 'my_custom_field' first. Use DESC to show posts with the field existing first. Use ASC to show posts with the field existing last. Use 'meta_value' instead if the custom field does not contain numeric values. 'title' => 'ASC' // Sort by post title A-Z ], 'meta_key' => 'my_custom_field' ] ]; return $options; }, 10, 2 );
Make sure to use meta_value
in line 24 if the custom field does not contain numeric values.
It’s important that the NOT EXISTS
comparison is before the EXISTS
comparison. See this StackExchange question for more info and examples.
Also be aware that ordering with named meta_query keys does not work in this setup.