JS objects and functions
FacetWP uses several different JS objects to store and handle data on the front-end: FWP, FWP_HTTP, and FWP_JSON. Each of these objects is described below.
Viewing the data
To view the data in each of these objects, type its name into the browser console:
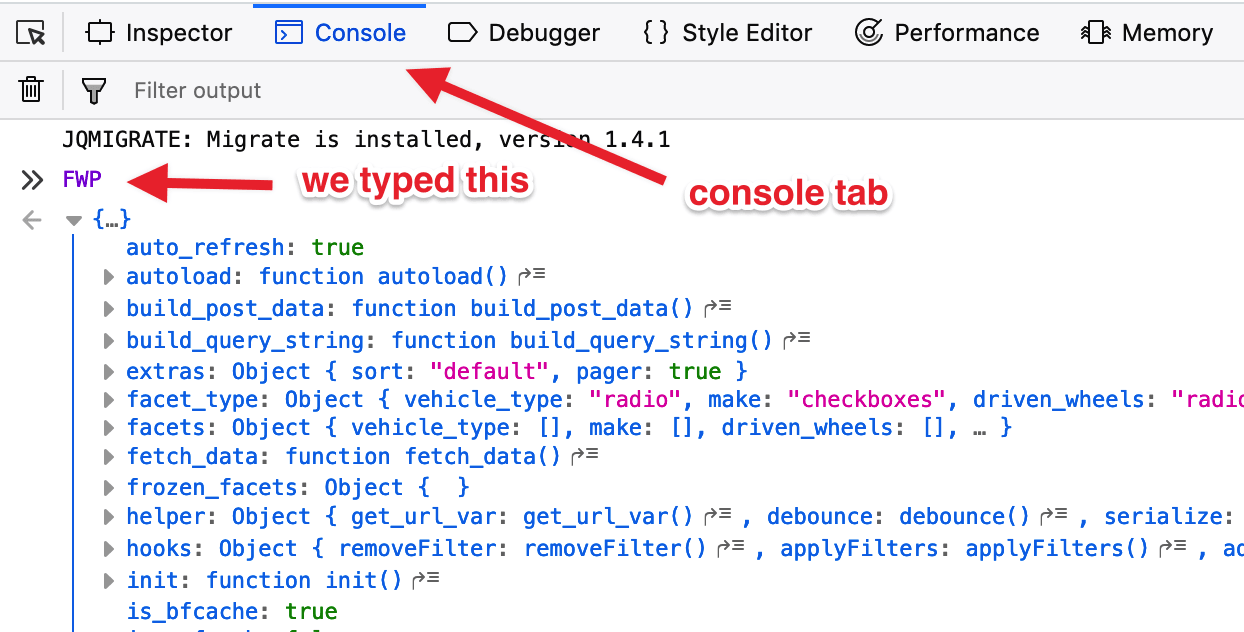
The data in these objects will change on each dynamic page load. So after each facet interaction you can check what the current status of the data is. This can be enormously helpful when developing custom code or debugging issues.
Here is a short explainer video to get you started:
FWP
The FWP
object contains the bulk of FacetWP’s data and logic. It contains a mixture of functions, variables, and data objects. Much of this is covered below.
Data objects
How to use custom JavaScript code?
JavaScript code can be placed in your (child) theme's main JavaScript file. Alternatively, you can add it manually between
<script>
tags in the<head>
section of your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More infoFWP.facets; // Contains each facet's selected values FWP.extras; // Determines which extra features to use (pager, sort, etc) FWP.hooks; // JS hook system, based on the WP_JS_Hooks library FWP.frozen_facets; // A low-level object to force certain facet types to only re-render when needed FWP.active_facet; // Stores information for the currently active, selected facet. Only available during the 'facetwp-refresh' event FWP.response; // Contains the AJAX response data FWP.settings; // Facet-specific settings, as well as some global data: FWP.settings.labels; // The names and labels of all facets on the page FWP.settings.num_choices; // The number of choices in all facets on the page FWP.settings.pager; // Pagination information: the current page, post per page, number of posts, number of pages
Functions
How to use custom JavaScript code?
JavaScript code can be placed in your (child) theme's main JavaScript file. Alternatively, you can add it manually between
<script>
tags in the<head>
section of your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More infoFWP.refresh(); // Parses the facets and triggers an ajax refresh FWP.reset(); // Resets all facets FWP.fetchData(); // Triggers an AJAX refresh without re-parsing the facets FWP.parseFacets(); // Applies any facet HTML changes to FWP.facets FWP.setHash(); // Updates the permalink URL FWP.loadFromHash(); // Populates facet data from URL data (happens on pageload) FWP.buildQueryString(); // Builds and returns the URL query string (without preceding questionmark) FWP.init(); // Initializes all FacetWP event listeners
Variables
How to use custom JavaScript code?
JavaScript code can be placed in your (child) theme's main JavaScript file. Alternatively, you can add it manually between
<script>
tags in the<head>
section of your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More infoFWP.auto_refresh = true; // Whether or not to auto-refresh when a facet choice is selected FWP.soft_refresh = false; // This is set to true when using the Pager facet (page numbers, per page and load more), and the old sort box. Skips processing of other facets. FWP.is_refresh = false; // This is set to true when a refresh is active FWP.is_reset = false; // This is set to true when resetting FWP.loaded = true; // This is false on the initial page load and true after the first "facetwp-loaded" JS event fires. It then remains true. FWP.paged // Used in pagination
FWP_HTTP
The FWP_HTTP
object contains data for the current page’s URI and its $_GET
variables.
How to use custom JavaScript code?
JavaScript code can be placed in your (child) theme's main JavaScript file. Alternatively, you can add it manually between
<script>
tags in the<head>
section of your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More infoFWP_HTTP.get; // an object containing the page's $_GET variables FWP_HTTP.uri; // the current page's URI (minus any beginning or trailing slashes)
FWP_JSON
The FWP_JSON
object contains generally static data, such as the endpoint URL (ajaxurl
) and the facet URL prefix. Here are examples of its data:
How to use custom JavaScript code?
JavaScript code can be placed in your (child) theme's main JavaScript file. Alternatively, you can add it manually between
<script>
tags in the<head>
section of your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More infoFWP_JSON.ajaxurl; // the current ajax endpoint URL FWP_JSON.no_results_text; // the text that appears for "No results found" screens FWP_JSON.nonce; // useful when making authenticated ajax requests FWP_JSON.prefix; // the prefix for each facet's URL variable, usually "_" or "fwp_"
Using FWP.reset()
Besides using a Reset facet, it is also possible to reset (all or some) facets programmatically, with the FWP.reset()
function:
Reset all facets programmatically
Reset all facets:
How to use custom JavaScript code?
JavaScript code can be placed in your (child) theme's main JavaScript file. Alternatively, you can add it manually between
<script>
tags in the<head>
section of your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More infoFWP.reset();
Reset all facets with a button:
<button onclick="FWP.reset()">Reset</button>
Or with a link:
<a href="javascript:;" onclick="FWP.reset()">Reset</a>
Paste the button or link into an HTML widget, HTML block, or directly into your PHP template file.
Reset some facets programmatically
Reset one facet:
How to use custom JavaScript code?
JavaScript code can be placed in your (child) theme's main JavaScript file. Alternatively, you can add it manually between
<script>
tags in the<head>
section of your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More infoFWP.reset('make');
Reset multiple facets:
How to use custom JavaScript code?
JavaScript code can be placed in your (child) theme's main JavaScript file. Alternatively, you can add it manually between
<script>
tags in the<head>
section of your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More infoFWP.reset(['make', 'model', 'year']);
Reset individual facet choices:
How to use custom JavaScript code?
JavaScript code can be placed in your (child) theme's main JavaScript file. Alternatively, you can add it manually between
<script>
tags in the<head>
section of your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More infoFWP.reset({ 'make': 'audi' });
Note that can also use FWP.facets within a facetwp-refresh event to (re)set facet values, if needed conditionally, based on other facet selections.
Using FWP.fetchData()
Set a facet value and trigger a refresh
Let’s say you want to programmatically set a facet value and trigger a refresh. Your gut might tell you to use FWP.refresh()
. Since this function parses the facets, it would actually overwrite the values you’re trying to set.
The following example shows how to do this, using FWP.facets to set the facet value, then using FWP.fetchData()
to trigger an AJAX refresh without re-parsing all facets:
How to use custom JavaScript code?
JavaScript code can be placed in your (child) theme's main JavaScript file. Alternatively, you can add it manually between
<script>
tags in the<head>
section of your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More infoFWP.facets['car_brand'] = ['audi']; FWP.fetchData(); FWP.setHash(); // Optional: update the URL
Note that can also use FWP.facets within a facetwp-refresh event to (re)set facet values, if needed conditionally, based on other facet selections.
Using FWP.active_facet
The FWP.active_facet
object contains info about the currently active facet, which is the facet that is currently used/clicked. The object is only available during the refresh process, so within the facetwp_refresh event, which gets triggered before FacetWP begins the refresh process.
Get the currently active facet’s name or type
The following example shows how to get the active facet’s name or type within the facetwp-refresh
event:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'facetwp_scripts', function() { ?> <script> document.addEventListener('facetwp-refresh', function() { // Runs before the refresh process, on each AJAX page load, and on the initial page load. if (null !== FWP.active_facet) { let facet = FWP.active_facet; let facet_name = facet.attr('data-name'); let facet_type = facet.attr('data-type'); // Do something console.log(facet_name); console.log(facet_type); } }); </script> <?php } );
Note that FWP.active_facet
contains no info about which facets or facet choices are currently selected. You can use the FWP.facets
object for that:
Using FWP.facets
The FWP.facets
object can be used to check (or set) a facet’s selected choice(s).
Check if a specific facet has any choice(s) selected
The following example uses the FWP.facets
object to check if a specific facet has any choice(s) selected:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_head', function() { ?> <script> document.addEventListener('facetwp-loaded', function() { // Runs after the refresh process, on each AJAX page load, and on the initial page load. let selected = FWP.facets['my_facet_name']; // Replace "my_facet_name" with the name of your facet if ( selected.length ) { // If any choice in this facet is selected // Do something } }); </script> <?php }, 100 );
Check if a specific facet has a specific choice selected
The following example uses the FWP.facets
object to check if a specific facet has a specific choice selected. The specified choice must be the indexed facet_value
, as it is displayed in the URL after selecting that choice.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_head', function() { ?> <script> document.addEventListener('facetwp-loaded', function() { // Runs after the refresh process, on each AJAX page load, and on the initial page load. let selected = FWP.facets['my_facet_name']; // Replace "my_facet_name" with the name of your facet if ( selected == 'my_facet_choice' ) { // If the choice "my_facet_choice" in this facet is selected // Do something } }); </script> <?php }, 100 );
To check if any facet has any choice(s) selected, you can use FWP.buildQueryString().
Output the number of active facets
The following example uses the FWP.facets
object to calculate and output the number of facets that are currently in use on the page. You can optionally exclude your Pager facet(s) and/or Sort facet(s) (or any other facet) in line 9:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
// Replace 'mypagerfacetname' and 'mysortfacetname' with the name of your Pager facet and Sort facet. // These facets will be excluded from the count. add_action( 'wp_head', function() { ?> <script> document.addEventListener('facetwp-loaded', function() { var count = 0; Object.entries(FWP.facets).forEach(([name, val]) => { if (name !== 'mypagerfacetname' && name !== 'mysortfacetname' && val.length > 0) { count ++; } }); // Do something with the count variable console.log( count ); // Outputs the number of facets in use to the Console. }); </script> <?php }, 100 );
Note that to get the currently active facet, at the moment that it is used/clicked, you can use FWP.active_facet.
Output the number of active facet choices
The following example uses the FWP.facets
object to calculate and output the number of facet choices that are currently selected on the page. You can optionally exclude your Pager facet(s) and/or Sort facet(s) (or any other facet) in line 9:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
// Replace 'mypagerfacetname' and 'mysortfacetname' with the name of your Pager facet and Sort facet. // These facets will be excluded from the count. add_action( 'wp_head', function() { ?> <script> document.addEventListener('facetwp-loaded', function() { var count = 0; Object.entries(FWP.facets).forEach(([name, val]) => { if (name !== 'mypagerfacetname' && name !== 'mysortfacetname' && val.length > 0) { count += val.length; } }); // Do something with the count variable console.log( count ); // Outputs the number of selected facet choices to the Console. }); </script> <?php }, 100 );
Note that to get the currently active facet, at the moment that it is used/clicked, you can use FWP.active_facet.
Using FWP.buildQueryString()
The FWP.buildQueryString()
function builds and returns the URL query string. It can be used to check if any facet choices have been selected:
Check if any facet has any choice(s) selected
The following example uses FWP.buildQueryString()
to check if any facet has any choice(s) selected, in which case the query string is not empty. This will work for any facet, except the Reset facet, which empties the query string.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_head', function() { ?> <script> document.addEventListener('facetwp-loaded', function() { // Runs after the refresh process, on each AJAX page load, and on the initial page load. if ( '' != FWP.buildQueryString() ) { // Run only when there are facet selections in the URL // Do something } }); </script> <?php }, 100 );
Using FWP.loaded
The FWP.loaded
variable can be used to determine if it is the initial page load or not. You can use it in a condition to let code run only once on the initial page load, or only after that, on each subsequent AJAX page load.
The way it works is that the variable is false
by default, then becomes (and remains) true
after the first facetwp-loaded JS event has fired, which is after FacetWP has finished the refresh process of the initial page load. See the testing code below which demonstrates this process.
Let code run only after the initial page load
The following example shows how to run code only after the initial page load, meaning on every subsequent AJAX page load triggered by facet interaction.
The following example does the same as the above example that checks if a specific facet has any choice(s) selected. But now, it only runs when FWP.loaded
is true
, which it is after any facet interaction. It will not run anymore on the initial page load, also not when there are facet choices in the URL.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_head', function() { ?> <script> document.addEventListener('facetwp-loaded', function() { // Runs after the refresh process, on each AJAX page load, and on the initial page load. if ( FWP.loaded ) { // Run after the initial page load let selected = FWP.facets['my_facet_name']; // Replace "my_facet_name" with the name of your facet if (selected.length) { // If any choice in this facet is selected // Do something } } }); </script> <?php }, 100 );
Let code run only on the initial page load
If you want a script to only run on the initial page load (only once), you can do the opposite: add !
(meaning “not”) before the variable: ! FWP.loaded
. This is only true
when the page loads the first time.
The following example does the same as the above example that checks if any facet has any choice(s) selected. But now, it only runs once, on the initial page load. In other words, this code checks if there are any facet choices in the URL when the page loads the first time. This can be useful to do something (like a page scroll) only after using a Submit Button, or after a redirect from a search box on another page.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_head', function() { ?> <script> document.addEventListener('facetwp-loaded', function() { // Runs after the refresh process, on each AJAX page load, and on the initial page load. if ( ! FWP.loaded ) { // Run on the initial page load only if ( '' != FWP.buildQueryString() ) { // Run only when there are facet selections in the URL // Do something } } }); </script> <?php }, 100 );
You can use FWP.loaded
both within the facetwp-refresh
and in the facetwp-loaded
events. The behavior is the same: it is false
on initial page load, true
after facet interaction, as the following script demonstrates:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_footer', function() { ?> <script> alert('initial: ' + FWP.loaded); // 'undefined' document.addEventListener('facetwp-refresh', function() { // Runs before the refresh process, on each AJAX page load, and on the initial page load. alert('refresh-1: ' + FWP.loaded); // 'false' on initial page load, 'true' after facet interaction if ( FWP.loaded ) { // Runs only after the first page load alert('refresh-2 ' + FWP.loaded); // true' after facet interaction } }); document.addEventListener('facetwp-loaded', function() { // Runs after the refresh process, on each AJAX page load, and on the initial page load. alert('loaded-1 ' + FWP.loaded); // 'false' on initial page load, 'true' after facet interaction if ( FWP.loaded ) { // Runs only after the first page load alert('loaded-2 ' + FWP.loaded); // 'true' after facet interaction } }); </script> <?php }, 100 );
Using FWP.auto_refresh and FWP.refresh()
One application of the FWP.refresh()
function is in an “Apply” or “Submit” button. FacetWP automatically refreshes whenever any interaction happens, but it’s possible to disable this if needed, by setting the FWP.auto_refresh
variable to false
. An “Apply” button triggering FWP.refresh()
can then be used to trigger the facet refresh. See this tutorial for a full explanation and code examples.
Using FWP.paged, FWP.soft_refresh and FWP.refresh()
If you have a use case for programmatically paginating with Javascript, you can do that like this:
How to use custom JavaScript code?
JavaScript code can be placed in your (child) theme's main JavaScript file. Alternatively, you can add it manually between
<script>
tags in the<head>
section of your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More infoFWP.paged = 3; // Set the page number FWP.soft_refresh = true; // Skip processing of other facets than the Pager facet FWP.refresh(); // Run a refresh
This would have to be used within the facetwp-refresh event. See this snippet and our code library for full examples.