facetwp_facets
Overview
With this hook, you can programmatically register/add facets, instead of using the plugin interface.
Why register facets programmatically?
Here are several reasons why you may want to register your facets in code:
- To prevent users from changing facet settings. Facets that are added this way will appear as “locked facets” in the plugin settings. While this does not absolutely prevent users from changing the facet settings, it will take a deliberate “unlock” action to do so (and this “unlock” action can be disabled too).
- You can have your facets and their settings under version control (e.g. with git). This way you can revert to previous sets of facets or previous facet settings.
- If your facets and settings are tied to a theme, anyone using the code/theme automatically has the registered facets and their settings.
- If you are using FacetWP with WordPress multi-site, all sites in the multi-site network can have the same facets and facet settings, as long as they are using the same theme. You no longer have to change facet settings in each site separately, and you can maintain them in one place: the theme’s functions.php file.
Parameters
- $facets | array | An array of existing facets
How to register a facet programmatically
To register one or more facets in code, you need to pass an array of the facet(s) settings to the hook, either in JSON, or in PHP, as shown below.
But how to get all available settings for a particular facet type? Every facet at least has a label
, name
, and type
. Most facet types have a source
. But the rest of the settings depend on the facet type.
You could check this (incomplete) overview. But the quickest way to get a complete and up-to-date array of all available settings for any given facet type is to first create the facet in the interface, set all its options, and then export the facet.
To do so, go to Settings > FacetWP > Settings > Import/Export, select one or more facets and click the “Export” button:
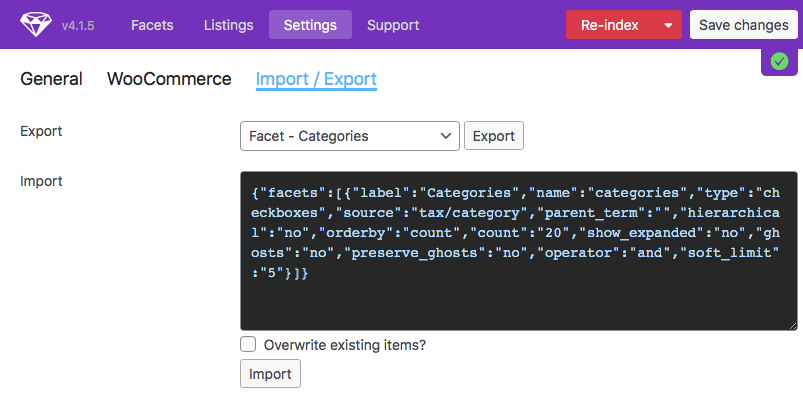
Copy the exported JSON code that appears in the text box. Make sure to keep the JSON safe, because the next step is to delete the facet(s) you just exported (use the gear icon, then click “Save changes”), otherwise the code-registered facet(s) will not appear.
Now you have two options to register the facet(s):
Using exported JSON directly
To use the exported JSON of one or more facets directly, you can add the following code to your (child) theme’s functions.php. Paste the exported JSON between the single quotes on line 5.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facets', function( $facets ) { // Paste the exported JSON between the single quotes like this: json_decode('PASTE JSON HERE'); $add_facets = json_decode( '{"facets":[{"enable_relevance":"yes","name":"my_search","label":"My Search","type":"search","search_engine":"","placeholder":"Enter keywords","auto_refresh":"no"}]}', true ); foreach ( $add_facets['facets'] AS $new_facet ) { $facets[] = $new_facet; } return $facets; });
After refreshing the page, your new code-registered facet(s) should appear in the list, at the bottom, with a “locked” icon. If you don’t see the locked facet(s), make sure you have deleted the facet(s) you exported (use the gear icon, then click “Save changes”), otherwise the code-registered facet(s) will not appear.
Using a converted PHP array
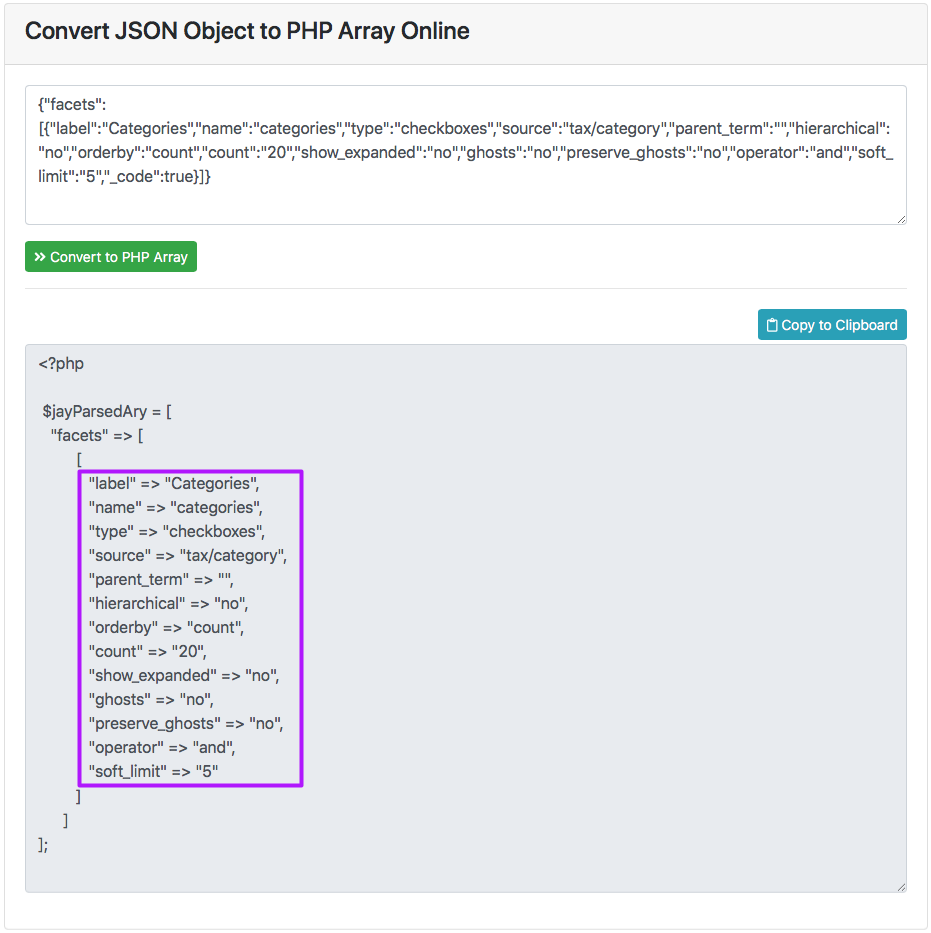
If you prefer seeing the facet settings array, or you want to make manual changes more easily, you can convert the exported JSON to a PHP array first.
A quick way to do this is to paste it into an online JSON to PHP array converter like https://jsontophp.com.
Copy the converted PHP array’s key/value pairs with all the facet’s settings and paste them into the hook code, as shown in the examples below.
Register one new facet
For example, to register a new Search facet named my_search
, add the following code to your (child) theme’s functions.php:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facets', function( $facets ) { // add a new 'my_search' facet to the existing $facets array $facets[] = [ // settings for this facet 'enable_relevance' => 'yes', 'name' => 'my_search', 'label' => 'My Search', 'type' => 'search', 'search_engine' => '', 'placeholder' => 'Enter keywords', 'auto_refresh' => 'no' ]; // return the modified $facets array return $facets; }, 10, 1 );
After refreshing the page, your new code-registered facet should appear in the list, at the bottom, with a “locked” icon. If you don’t see the locked facet, make sure you have deleted the facet you exported (use the gear icon, then click “Save changes”), otherwise the code-registered facet will not appear.
Register multiple new facets
To register multiple facets at once, just add another facet to the $facets
array. The following example adds two new facets: a Checkboxes facet named categories
and a Search facet named my_search
:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facets', function( $facets ) { // add a new 'categories' facet to the existing $facets array $facets[] = [ // settings for this facet 'label' => 'Categories', 'name' => 'categories', 'type' => 'checkboxes', 'source' => 'tax/category', 'parent_term' => '', 'hierarchical' => 'no', 'orderby' => 'count', 'count' => '20', 'show_expanded' => 'no', 'ghosts' => 'no', 'preserve_ghosts' => 'no', 'operator' => 'and', 'soft_limit' => '5' ]; // add a new 'my_search' facet to the existing $facets array $facets[] = [ // settings for this facet 'enable_relevance' => 'yes', 'name' => 'my_search', 'label' => 'My Search', 'type' => 'search', 'search_engine' => '', 'placeholder' => 'Enter keywords', 'auto_refresh' => 'no' ]; // return the modified $facets array return $facets; }, 10, 1 );
After refreshing the page, your new code-registered facets should appear in the list, at the bottom, with a “locked” icon. If you don’t see the locked facets, make sure you have deleted the facets you exported (use the gear icon, then click “Save changes”), otherwise the code-registered facets will not appear.
Locked facets
After registering a facet with this hook, it will appear in FacetWP’s Facets overview with a “locked” icon:
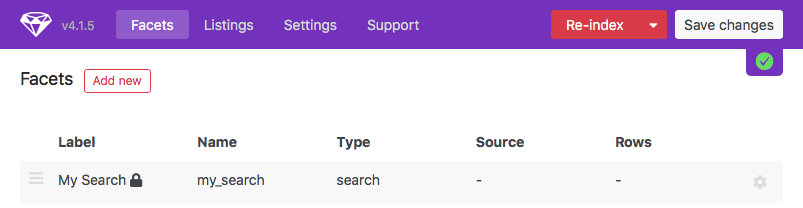
If the new facet does not appear, make sure its name is unique and not already used for another facet. Make sure you have deleted the facet(s) you exported (use the gear icon, then click “Save changes”), otherwise the code-registered facet(s) will not appear.
The settings screen of a locked facet will have a red “This facet is registered in code. Click to allow edits” warning on top, and the settings will be dimmed and become unclickable:
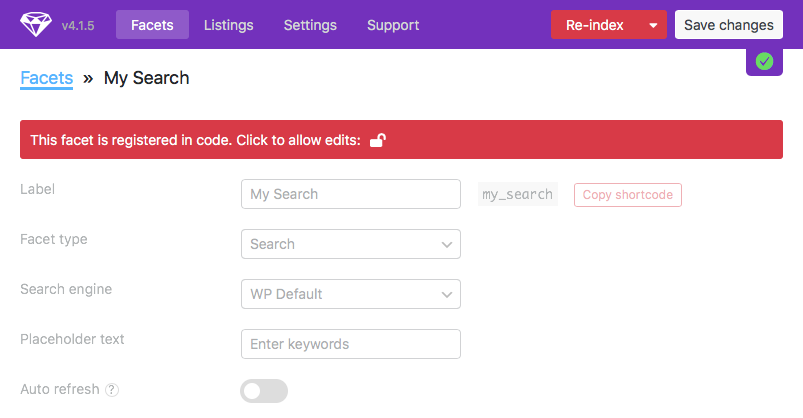
When you click the lock icon behind “Click to allow edits”, and then change the settings (or leave the settings as they are) and click “Save changes”, the facet will no longer be registered by your code, but again in the database like a normal facet. After that, changes to the facet settings in the hook will no longer have an effect unless you change the facet’s name in the code, which will then create a new code-registered facet with that name.
If you want to prevent other users from unlocking facets this way, you’ll have to register them in a slightly different way:
Prevent unlocking of facets
If you are registering your facets in code, and want to prevent admin users from unlocking facets (accidentally or on purpose), you can register your facet(s) as follows. The extra code makes sure that unlocked code-registered facets are deleted and added again as a locked facet, basically preventing them from being changed or removed.
Don’t forget to add the extra CSS that removes the “Click to allow edits” link.
To register facets directly with JSON, use the following:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facets', function( $facets ) { $add_facets = json_decode( '{"facets":[{"enable_relevance":"yes","name":"my_search","label":"My Search","type":"search","search_engine":"","placeholder":"Enter keywords","auto_refresh":"no"}]}', true ); $adding = wp_list_pluck( $add_facets['facets'], 'name' ); foreach ( $facets AS $facet => $values ) { if ( in_array( $values['name'], $adding ) && !isset( $values['_code'] ) ) { unset( $facets[$facet] ); // Unset unlocked code-based facets } } foreach ( $add_facets['facets'] AS $new_facet ) { $facets[] = array_merge( $new_facet, [ '_code' => true ] ); // Re-lock facets if previously unlocked } return $facets; });
Or, to register facets with a PHP array:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_facets', function( $facets ) { $add_facets = [ 'facets' => [ [ // settings for this facet 'label' => 'Categories', 'name' => 'categories', 'type' => 'checkboxes', 'source' => 'tax/category', 'parent_term' => '', 'hierarchical' => 'no', 'orderby' => 'count', 'count' => '20', 'show_expanded' => 'no', 'ghosts' => 'no', 'preserve_ghosts' => 'no', 'operator' => 'and', 'soft_limit' => '5' ], [ // settings for this facet 'enable_relevance' => 'yes', 'name' => 'my_search', 'label' => 'My Search', 'type' => 'search', 'search_engine' => '', 'placeholder' => 'Enter keywords', 'auto_refresh' => 'no' ] ] ]; $adding = wp_list_pluck( $add_facets['facets'], 'name' ); foreach ( $facets AS $facet => $values ) { if ( in_array( $values['name'], $adding ) && !isset( $values['_code'] ) ) { unset( $facets[$facet] ); // Unset unlocked code-based facets } } foreach ( $add_facets['facets'] AS $new_facet ) { $facets[] = array_merge( $new_facet, [ '_code' => true ] ); // Re-lock facets if previously unlocked } return $facets; });
Next, add the following CSS, which makes sure there is no way to edit the locked settings. The “Click to allow edits” link will be removed, and a “This facet is registered in code. It cannot be edited.” will show:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action('admin_head', function() { echo '<style> #app .facetwp-region .item-locked { display: none; } #app .facetwp-region-facets .locked:before { content: "This facet is registered in code. It cannot be edited."; display: block; padding: 10px; margin-bottom: 20px; background-color: var(--red); border-radius: 3px; color: var(--white); position: relative; z-index: 100000; } </style>'; });
With this CSS the red “locked” banner will look like this:
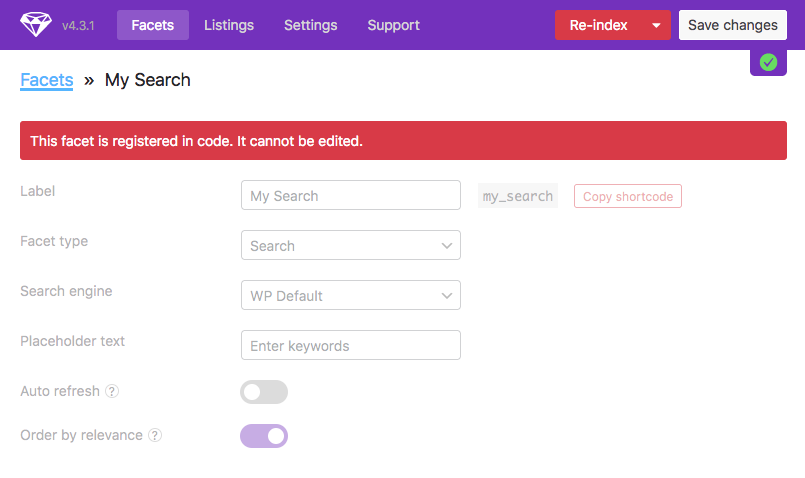