How to use custom code?
Our Help Center articles and our custom code library contain a lot of code snippets to add or customize FacetWP functionality.
On this page, intended for beginners, we’ll explain where and how to use these code snippets, for each type of code: PHP, JavaScript and jQuery, CSS, and shortcodes. In the Help Center, the type of code can be found in the badge in the top right corner of each code block.
Using PHP code snippets
PHP code can be added in the following three ways:
Add PHP code to your functions.php
The most straightforward way is to add the PHP code to your (child) theme’s functions.php
file. Copy the code, paste it at the bottom of the file, adapt it as needed, and upload it.
There are a few downsides of using functions.php
:
- If you make an error, your site will likely crash and may become inaccessible. If this happens, you’ll have to manually fix the mistake or remove the custom code to regain access to your site.
- If you ever decide to switch themes, your custom code will not be used and you have to manually move it to the new theme’s
functions.php
file. - If you upgrade your theme and aren’t using a child theme, all your custom code will be lost. Make sure to always have backups of your (child) theme files to prevent this.
These downsides can be overcome by using the Custom Hooks add-on:
Add PHP code with the Custom Hooks add-on
If you are hesitant to touch your (child) theme’s functions.php
file because of the downsides mentioned above, or if you don’t have access to it, you can use the Custom Hooks add-on. This plugin is basically an empty PHP file in which you can paste custom PHP snippets. To use it, follow these steps:
- Download the Custom Hooks add-on from your account page.
- Upload it to your
wp-content/plugins
folder and activate the plugin. - In your site dashboard, browse to
Plugins > Plugin File Editor
. If you don’t see it, see below. - Select “Custom Hooks” from the plugin dropdown (above the code editor), and click “Select”.
- Paste relevant code into
custom-hooks.php
, then click “Update File”. - Optionally, download the changed
custom-hooks.php
file as a backup.
As an alternative to using the Plugin File Editor in WordPress, you can also manually paste the code into plugins > custom-hooks > custom-hooks.php
and upload it with FTP.
Advantages of using the Custom Hooks add-on
The advantages of using the Custom Hooks add-on are:
- All your custom code is in one place, not mixed with theme code.
- For testing, you can disable all customizations by simply deactivating the plugin.
- Your custom code cannot be overwritten. This add-on will never receive updates.
- If you make a mistake that triggers a PHP fatal error, WordPress will simply disable the plugin and will not crash your site.
Enable the Plugin File Editor to edit the Custom Hooks add-on
In newer WordPress versions, the Plugin- and Theme File Editor is enabled by default, but sometimes it may be disabled.
You can enable it in wp-config.php
:
Search for define('DISALLOW_FILE_EDIT', true);
and replace it with:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
define('DISALLOW_FILE_EDIT', false);
Now the following options will be available: Plugins > Plugin File Editor, and Appearance > Theme File Editor.
Add PHP code with a code snippets plugin
Another way of adding custom PHP code is with a code snippets plugin. The most used are WPCode Lite and Code Snippets.
Besides ease of use, the advantage of using a code snippets plugin is that each snippet is contained in its own item that can be disabled, edited, exported and deleted with a click. You can easily add a description to remind you of what it does, change the priority at which the snippet’s hook runs, and limit where the code runs (e.g. only in the front-end).
Using JavaScript code snippets
If the custom code is enclosed in <script>
tags, then it’s JavaScript code. Or to be precise: everything within the <script>
tags is JavaScript code, while the whole snippet is HTML (as <script>
tags are HTML). You may also see plain JavaScript snippets without the <script>
tags.
Many code examples are written in jQuery, which also is JavaScript. These snippets need the jQuery library loaded. If it is not already loaded in your theme, you can enable jQuery in FacetWP’s settings.
You’ll also encounter scripts that use fUtil. fUtil is a small JavaScript helper library that comes built-in with FacetWP. Its functionality is similar to jQuery, with the difference that fUtil relies entirely on modern, vanilla JavaScript. It can be used as a lightweight substitute on websites that don’t need or load jQuery. FacetWP itself does not need jQuery to function, and you can disable loading it in FacetWP’s settings if your theme or other plugins do not need it.
Add JavaScript code to your main JS file or inline
If you know what you are doing, you can add the plain JavaScript code directly to your (child) theme’s linked main JavaScript file (without the <script>
tags):
How to use custom JavaScript code?
JavaScript code can be placed in your (child) theme's main JavaScript file. Alternatively, you can add it manually between
<script>
tags in the<head>
section of your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More infodocument.addEventListener('facetwp-loaded', function() { fUtil('.facetwp-checkbox.checked .facetwp-expand').trigger('click'); }
Or you can manually add it inline to the <head>
or footer section of your website, enclosed in <script>
tags. The <head>
section can generally be found in your theme’s header.php
file, and the footer section in footer.php
.
<script> // The actual JavaScript starts here document.addEventListener('facetwp-loaded', function() { fUtil('.facetwp-checkbox.checked .facetwp-expand').trigger('click'); }); </script>
You can also add JavaScript to the header or footer with a PHP hook:
Add JavaScript code with a PHP hook
If you add custom JavaScript code directly to the page (instead of in a linked file), it needs to run either in the <head>
or in the footer section of your website.
WordPress has two hooks that can inject all kinds of code in the header and footer: wp_head
for for the head section and wp_footer
for the footer section.
Just like any other PHP snippet, these PHP hooks can be added to your site in the following three ways:
Add JavaScript code to the head section with a hook
To add Javascript code to the <head>
of all of your site’s pages, you can use the wp_head hook, which runs in header.php
:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_head', function () { ?> <script> // add your JavaScript code here </script> <?php }, 100 );
Here is a full example with the different parts:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
// This is PHP that runs in header.php add_action( 'wp_head', function() { ?> <!-- This is the HTML injected in the <head> section --> <script> // The actual JavaScript starts here (function($) { $(document).on('facetwp-refresh', function() { $('.facetwp-template').animate({ opacity: 0 }, 1000); }); $(document).on('facetwp-loaded', function() { $('.facetwp-template').animate({ opacity: 1 }, 1000); }); })(jQuery); </script> <?php }, 100 ); // 100 is the hook's priority
The whole hook – now a PHP code snippet – can be added to your site in one of the above three ways.
Note: the 100
in the last line is the so-called “priority”. The priority determines the order in which this particular action hook runs, if there is more than one. Lower numbers correspond with earlier execution. The default priority is 10
, so 100
makes it run late, after other hooks. Sometimes you’ll see specific priorities that make the hook run before or after certain other hooks have run.
Add JavaScript code to the footer section with a hook
Sometimes custom JavaScript code needs to run after the page has fully loaded, after all other JavaScript. In that case, the code needs to be added to the page footer.
Depending on the code, this can be done in two ways: using FacetWP’s facetwp_scripts
hook, or using WP’s wp_footer
hook:
Using the facetwp_scripts hook
If your JavaScript code needs to run only on pages where FacetWP is active (pages with facets), you can use FacetWP’s facetwp_scripts hook.
This hook is an alternative to using the wp_footer hook, which runs on all pages.
The facetwp_scripts
hook usually is the safest choice for FacetWP-related JavaScript. If you would use the wp_footer
hook to inject custom code that contains JavaScript that is dependent on code that is loaded only on pages where FacetWP is active, like FacetWP’s fUtil library, you’ll get JavaScript errors on pages where FacetWP is not loaded. Using facetwp_scripts
instead prevents these errors.
To add code to the footer of all pages with facets, use:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'facetwp_scripts', function () { ?> <script> // Add your JavaScript code here </script> <?php }, 100 );
Here is a full example:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
// This is PHP that runs in the footer section add_action( 'facetwp_scripts', function() { ?> <!-- This is the HTML injected in the footer section, only on pages where FacetWP is active --> <script> (function($) { document.addEventListener('facetwp-refresh', function() { if ( FWP.loaded ) { $( '.facetwp-template' ).addClass( 'template-loading' ); } }); document.addEventListener('facetwp-loaded', function() { $( '.facetwp-template' ).removeClass( 'template-loading' ); }); })(fUtil); </script> <?php }, 100 ); // 100 is the hook's priority
The whole hook – now a PHP code snippet – can be added to your site in one of the above three ways.
Using the wp_footer hook
If your JavaScript code needs to run on all pages of your site, you need to use the wp_footer hook, which also runs on all pages. It looks and works the same as the wp_head hook:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_footer', function () { ?> <script> // Add your JavaScript code here </script> <?php }, 100 );
The whole hook – now a PHP code snippet – can be added to your site in one of the above three ways.
Using CSS code snippets
If the custom code is enclosed in <style>
tags, then it’s CSS code. Or to be precise: everything within the <style>
tags is CSS code, while the whole snippet is HTML (as <style>
tags are HTML). You may also see plain CSS snippets (without <style>
tags).
Add CSS code to your theme’s CSS file or inline
If you know what you are doing, you can add the CSS code directly to your (child) theme’s linked main style.css
file (without the <style>
tags):
How to use custom CSS?
CSS code can be placed in your (child) theme's style.css file. Alternatively, you can add it manually between
<style>
tags in the<head>
section, in your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More info.facetwp-counter { font-size: 14px; font-style: italic; color: #888; }
Or you can manually add it inline to the <head>
section of your website, enclosed in <style>
tags. The <head>
section can generally be found in your theme’s header.php
file.
<style> /* The actual CSS starts here */ .facetwp-counter { font-size: 14px; font-style: italic; color: #888; } </style>
You can also add CSS to the header with a PHP hook:
Add CSS code to the head section with a hook
In general, if you add custom CSS code directly in the website’s header code (instead of in the linked style.css
file), it needs to be added to the <head>
section to be available for each page. To do so, you can add it manually to your (child) theme’s header.php
(enclosed in <style>
tags). Or you can use WordPress’ wp_head
PHP hook, which runs in header.php
:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_head', function () { ?> <style> /* Add your CSS code here */ </style> <?php }, 100 );
Here is a full example with the different parts:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
// This is PHP that runs in header.php add_action( 'wp_head', function () { ?> <!-- This is the HTML injected in the <head> section --> <style> /* The actual CSS starts here */ .facetwp-counter { font-size: 14px; font-style: italic; color: #888; } </style> <?php }, 100 ); // 100 is the hook's priority
Note: the 100
in the last line is the so-called “priority”. The priority determines the order in which this particular action hook runs, if there is more than one. Lower numbers correspond with earlier execution. The default priority is 10
, so 100
makes it run late, after other hooks. Sometimes you’ll see specific priorities that make the hook run before or after certain other hooks have run.
Just like any other PHP snippet, this PHP hook can be added to your site in the following three ways:
Combine Javascript and CSS code snippets in one hook
Some customizations need both JavaScript and CSS code. If the Javascript can run in the <head>
section, you can combine the JavaScript and the CSS in one wp_head
hook:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'wp_head', function () { ?> <style> /* Add your CSS code here */ </style> <script> // Add your JavaScript code here </script> <?php }, 100 );
If the JavaScript code needs to run in the footer, you could combine the CSS and JavaScript in a facetwp_scripts hook or a wp_footer hook. Or you could use one of these for the JavaScript in the footer and a separate wp_head
hook for the CSS, as CSS is usually best placed in the <head>
section.
Using shortcodes
If you see a short piece of code in square brackets, it’s likely a shortcode:
How to use shortcodes?
Shortcodes can be placed directly in post/page edit screens. You can also add them in text/HTML widgets. The WordPress Block Editor has a Shortcode block to place them in. And most Page builders have a dedicated shortcode module/widget. In PHP templates, shortcodes can be displayed with WP's do_shortcode() function:
echo do_shortcode('[my-shortcode]');
. More info[my-shortcode]
Shortcodes are a compact way to add custom PHP code that will run when the shortcode is parsed. Shortcodes are often already defined in a theme or plugin, ready to be used. But you can also create your own shortcodes, using WordPress’ add_shortcode function.
FacetWP comes with its own set of shortcodes. Each facet and Listing Builder listing you create, gets its own [facetwp]
shortcode that outputs the facet or listing. Also other FacetWP features can be placed with shortcodes.
Shortcodes often have attributes. For example, FacetWP shortcodes for facets have a facet
attribute that contains the facet name:
How to use shortcodes?
Shortcodes can be placed directly in post/page edit screens. You can also add them in text/HTML widgets. The WordPress Block Editor has a Shortcode block to place them in. And most Page builders have a dedicated shortcode module/widget. In PHP templates, shortcodes can be displayed with WP's do_shortcode() function:
echo do_shortcode('[my-shortcode]');
. More info[facetwp facet="the_facet_name"]
And shortcodes for listings have a template
attribute that contains the listing name:
How to use shortcodes?
Shortcodes can be placed directly in post/page edit screens. You can also add them in text/HTML widgets. The WordPress Block Editor has a Shortcode block to place them in. And most Page builders have a dedicated shortcode module/widget. In PHP templates, shortcodes can be displayed with WP's do_shortcode() function:
echo do_shortcode('[my-shortcode]');
. More info[facetwp template="the_listing_name"]
Shortcode placement
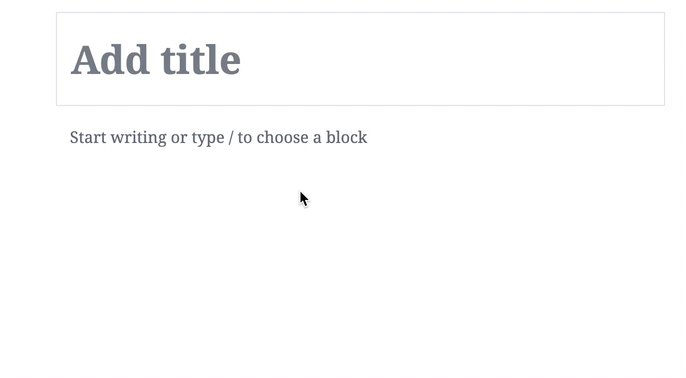
Shortcodes can be placed directly in post/page edit screens (in visual or code editor mode). You can also add them in text/HTML widgets (in Appearance > Widgets).
The WordPress Block Editor has a Shortcode block to place them in. And most Page builders have a dedicated shortcode module/widget.
You can also output shortcodes directly in your PHP templates, with WordPress’ do_shortcode() function:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
echo do_shortcode('[my-shortcode]');