How to pre-select facet choices
It is possible to pre-select facet choices on page load, using the facetwp_preload_url_vars hook.
For example, to pre-select audi
in the ‘make’ facet if the current page URI is demo/cars
and the facet isn’t already in use, add the following code to your (child) theme’s functions.php:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_preload_url_vars', function( $url_vars ) { if ( 'demo/cars' == FWP()->helper->get_uri() ) { // Replace 'demo/cars' with the URI of your page (everything after the domain name, excluding any slashes at the beginning and end) if ( empty( $url_vars['make'] ) ) { // Replace 'make' with the name of your facet $url_vars['make'] = [ 'audi' ]; // Replace 'audi' with the facet choice that needs to be pre-selected. Use the technical name/slug as it appears in the URL when filtering } } return $url_vars; } );
When a user visits http://yoursite.com/demo/cars/
, ‘Audi’ will now be pre-selected in the ‘make’ facet.
Two important things to keep mind when using the above code:
- The page URI is everything after the domain name without the URL variables, and without any slashes at the beginning and end.
- For the facet value you need to use its technical name/slug as it appears in the URL when filtering with that facet, not its display value you see as choice in the facet itself.
Setting the correct value for each facet type
Depending on the facet type, the pre-selected value may need to be a multi-element array. For each facet type you can see if this is the case by looking at the URL variables that are present after making a facet selection with that facet type. Some examples:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
// Date Range facet: $url_vars['your_date_field'] = ['2018-01-01', '2018-12-31']; // Number Range or Slider facet: $url_vars['your_number_field'] = ['25', '500']; // Proximity facet (lat, long, radius, label): $url_vars['your_proximity_field'] = ['38.9072', '-77.0369', '10', 'Washington%2C%20DC'];
Pre-select a facet choice for a category, tag, or taxonomy term archive
A common scenario is that you have a category, tag, or taxonomy term archive page, with a facet that uses the corresponding category/taxonomy as its source. Wouldn’t it be nice if that facet would automatically pre-select the current term’s corresponding choice?
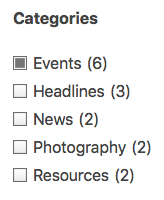
For example, say you have a category archive page /category/events/
, on which you have a facet named ‘categories’ which has categories (technically the ‘category’ taxonomy) as source, and you want the choice “events” to be pre-selected automatically.
In case you are using a Listing Builder listing template you may already be using the facetwp_template_use_archive hook to automatically pre-filter results based on the current category/tag/taxonomy term. But also this hook does not pre-select the facet choice that corresponds to the current category/tag/term archive’s term.
With the following code you can pre-select the current term’s corresponding choice in the facet. Adapt the facet name and taxonomy slug, and add it to your (child) theme’s functions.php:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php // Change 'categories' to the name of the categories/terms facet (2x) add_filter( 'facetwp_preload_url_vars', function( $url_vars ) { if ( false !== strpos( FWP()->helper->get_uri(), 'category' ) ) { // change 'category' whatever the main URL slug is for the taxonomy term archive if ( empty( $url_vars['categories'] ) ) { $term = basename( FWP()->helper->get_uri() ); $url_vars['categories'] = [$term]; } } return $url_vars; } );
Note that this code will only work when the category/tag/term slug (events
in this example) is the same as the facet value (which can be seen in the URL when you select the facet choice: /category/events/?categories=events
). The slug also needs to be the last part of the URI.
Pre-select a facet choice based on a custom field value
If you have facets on a normal post or page, and you need to pre-select a facet choice based on a value set in a custom field on that post or page, you may run into trouble getting the custom field’s value.
To get the value you can use get_post_meta(), or get_field() if you are using Advanced Custom Fields. In these functions, you need to pass the post_id
. Normally you’d get that with get_the_ID()
. However, this hook runs so early in WP’s execution that get_the_ID()
will return nothing.
The following example shows a way to do this, by using WP’s url_to_postid() function:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_preload_url_vars', function( $url_vars ) { if ( 'demo/cars' == FWP()->helper->get_uri() ) { // Replace 'demo/cars' with the URI of your page (everything after the domain name, excluding any slashes at the beginning and end) // Get the post ID from the URI $post_id = url_to_postid( FWP()->helper->get_uri() ); // Get the (ACF) custom field value // Use get_post_meta() if your field is not an ACF field $facet_value = get_field( 'page_field', $post_id, true ); if ( $facet_value && empty( $url_vars['make'] ) ) { // Replace 'make' with the name of your facet $url_vars['make'] = [ $facet_value ]; } } return $url_vars; } );
Note that if you have a multi-lingual site, the url_to_postid()
function will not properly return the post_id
, due to the language prefix in the URI. If this is the case, you can strip the language from the URI as follows (this replaces line 6 in above code example):
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
// Get the URI $uri = FWP()->helper->get_uri(); // Possible language prefixes in the URI $prefixes = array( 'en/', 'fr/', 'de/' ); // Strip language prefixes from the URI foreach ($prefixes as $prefix) { if (substr($uri, 0, strlen($prefix)) === $prefix) { $uri = substr($uri, strlen($prefix)); break; // Exit the loop when a matching prefix is found } } // get the post ID from the URI $post_id = url_to_postid( $uri );
(P)re-select a facet choice after a reset
If you are using a Reset facet, the pre-selected choice will be deselected again after a reset.
The following code re-selects that choice again when a user clicks the Reset button/link:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php add_action( 'wp_footer', function() { ?> <script> document.addEventListener('facetwp-loaded', function() { if (! FWP.loaded) { FWP.hooks.addAction('facetwp/reset', function() { FWP.facets['make'] = ['audi']; }); } }); </script> <?php }, 100 );
If you are using the above code example to pre-select a facet choice for a category, tag, or taxonomy term archive, this will not work because the facet choice to be pre-selected is different on each term archive page. You can use the following code instead:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php add_action( 'wp_footer', function() { ?> <script> document.addEventListener('facetwp-loaded', function() { if (! FWP.loaded && false !== FWP_HTTP.uri.indexOf('category')) { // change 'category' whatever the main URL slug is for the taxonomy term archive let term = FWP_HTTP.uri.split('/').reverse()[0]; FWP.hooks.addAction('facetwp/reset', function() { FWP.facets['categories'] = [term]; // Change 'categories' to the name of the categories/terms facet }); } }); </script> <?php }, 100 );
(P)re-select a facet choice when all other choices are deselected
If you want the pre-selected choice to be reselected again when all other choices are deselected in that facet, add the following to your (child) theme’s functions.php:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php add_action( 'wp_footer', function() { ?> <script> document.addEventListener('facetwp-refresh', function() { if ('undefined' !== typeof FWP.facets['make'] && FWP.facets['make'].length === 0) { // Replace 'make' with the name of your facet. FWP.facets['make'] = ['audi']; // Replace 'make' with the name of your facet, and 'audi' with the facet choice that needs to be pre-selected. Use the technical name/slug as it appears in the URL when filtering. } }); </script> <?php }, 100 );
Combined with the code at the beginning of this tutorial, this will basically keep the choice always selected as the default state, when no other options are selected for that facet.
Pre-select a Sort facet choice
If your post listing query has a specific order set with the “order” and “orderby” query arguments, you may want to let your Sort facet reflect that same order on page load. You can do this with the facetwp_preload_url_vars
hook.
Say your query is ordering by Post Title (A-Z) like this:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php $args = [ "post_type" => ["post"], "posts_per_page" => 10, "orderby" => ["title" => "ASC"], // Order by Post Title Ascending (A-Z) // ... your arguments ];
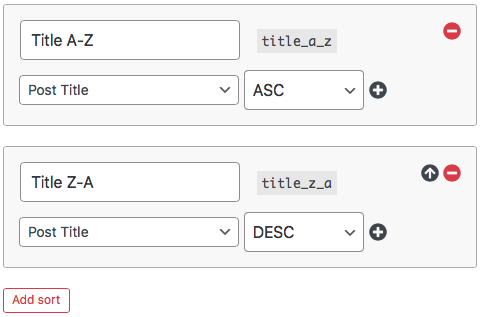
If your Sort facet has that same order set in a sort option called title_a_z
, like in the image on the right, you can now pre-select that option with the following code in your (child) theme’s functions.php:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php // Replace 'my/page' with the URI of your page (everything after the domain name, excluding any slashes at the beginning and end) // Replace 'my_sort_facet' with the name of your Sort facet and 'title_a_z' with the Sort facet option to be pre-selected add_filter( 'facetwp_preload_url_vars', function( $url_vars ) { if ( 'my/page' == FWP()->helper->get_uri() ) { if ( empty( $url_vars['my_sort_facet'] ) ) { $url_vars['my_sort_facet'] = ['title_a_z']; } } return $url_vars; } );