Customize marker clustering
This page gives an overview of possible customizations of the built-in marker clustering feature of the Map facet.
What is marker clustering?
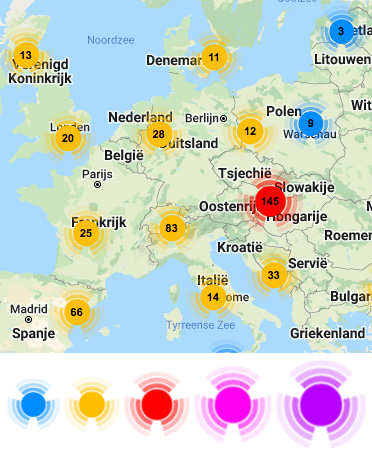
If you have large numbers of markers concentrated in specific regions, marker clustering can help simplify their display by grouping them into clusters.
Clusters will have one of five cluster icons, based on the total number of markers contained in them. Each cluster icon on the map will show the number of clustered markers in it. Clusters will “de-cluster” into their contained regular markers at specified zoom levels, and/or after clicking the cluster.
Marker clustering can be enabled with the “Marker clustering” setting in your Map facet.
The Gmaps Marker Clusterer JavaScript library used by FacetWP, and its options, methods and examples can be found here.
Set marker cluster to zoom in when clicking on it
By default, the map will not zoom in when clicking on a cluster. To let the map zoom into a cluster on click, add the following snippet to your (child( theme’s functions.php:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { if ( isset( $args['config']['cluster'] ) ) { $args['config']['cluster']['zoomOnClick'] = true; // default: false } return $args; } );
Set the maximum zoom level for a cluster to appear
The maximum zoom level that a marker can be part of a cluster can be set like this:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { if ( isset( $args['config']['cluster'] ) ) { $args['config']['cluster']['maxZoom'] = 10; // default: 15. Level must be between 1 and 20. } return $args; } );
Set the minimum number of markers in a cluster
The minimum number of markers to be in a cluster before the markers are hidden and a count is shown can be set like this:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { if ( isset( $args['config']['cluster'] ) ) { $args['config']['cluster']['minimumClusterSize'] = 5; // default: 2 } return $args; } );
Customize the marker cluster images
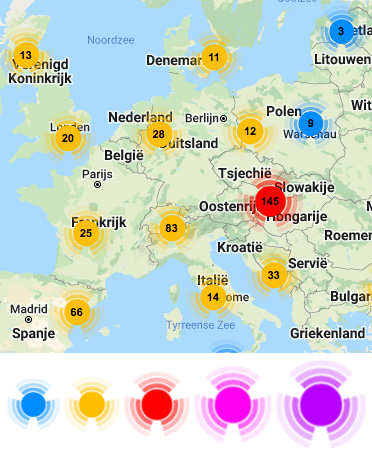
The Gmaps Marker Clusterer library used by FacetWP comes with five PNG cluster icon images, in ascending specific sizes.
The cluster icons’ sizes and colors represent the number of markers in the cluster. Which of the five cluster icons is shown, is calculated based on the number of markers in that specific cluster. From the smallest to the largest icon, the conditions to show that icon are: <10
, <100
, <1000
, <10000
, and >=10000
markers.
With the two methods described below, it is possible to change the default cluster images and their sizes.
Using the second method, you can also change the icon number’s text size and text color (which is black by default).
Method 1: Using imagePath and imageExtension
To simply replace the five default marker cluster icons with your own icons, use the following code:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { if ( isset( $args['config']['cluster'] ) ) { $args['config']['cluster']['imagePath'] = get_stylesheet_directory_uri() . '/assets/img/googlemaps/m'; // set your own image directory in your theme here. Note: the /m at the end is not a directory, but the first part of the image names. $args['config']['cluster']['imageExtension'] = 'png'; // 'svg' will work too } return $args; } );
For this to work, you need to make a custom directory in your theme. In the above example, this directory would be /assets/img/googlemaps
. The /m
in the specified directory is the first part of the image name.
In this example, the five images need the following specific names and sizes to work:
m1.png (size: 53x53 px, default color: blue) m2.png (size: 56x56 px, default color: yellow) m3.png (size: 66x66 px, default color: red) m4.png (size: 78x78 px, default color: magenta) m5.png (size: 90x90 px, default color: purple)
Note: You can use SVGs instead of PNGs. Just change imageExtension
to 'svg'
.
Method 2: Using the styles array
If you need more control, instead of using the imagePath
and imageExtension
options, you can use the styles array. With this method, you can not only set custom cluster images and sizes, but also change the cluster icons’ text color and text sizes.
The following example uses the default icon directory and icon sizes. It sets the cluster icon text (the number of markers in the cluster) to a size of 16px, and the color to #ffffff (white).
If you want to use custom icons and sizes, you can specify a directory in your theme with the 'url'
option, and place five images in it (see the previous example). Note that the /m
in the specified directory is not a directory but the first part of the image name. You can specify five custom icon sizes in the $clustericonsizes
array.
The two-dimensional styles
array needs five child arrays, for each of the five marker cluster icons, in ascending sizes:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $settings ) { $clustericonsizes = array( 53, 56, 66, 78, 90 ); // specify icon image sizes. These are the default values. for ( $i = 1; $i <= 5; $i ++ ) { $settings['config']['cluster']['styles'][] = [ 'url' => FACETWP_MAP_URL . '/assets/img/m' . $i . '.png', // directory, required. This is the default directory. The url becomes the CSS background-image url. 'width' => $clustericonsizes[ $i - 1 ], // required 'height' => $clustericonsizes[ $i - 1 ], // required 'textColor' => '#ffffff', // white, optional 'textSize' => 16 // 16px, optional // 'anchor' => [10,20], // Optional. Anchor position of the label text. By default label text is centered horizontally and vertically in the icon. [10,20] means 10px from the top of the icon (y) and 20px (x) from the left of the icon. Values need to be smaller than width and height of the icon to take effect. // 'iconAnchor' => [10,20], // Optional. The negative anchor position of the icon. [10,20] means the left top of the icon is 10px left of the lat/lng center, and 20px above the lat/lng center. Without iconAnchor values, the icon is centered on the lat/lng center horizontally and vertically. // 'backgroundPosition' => '5px 10px' // Optional. The CSS background-position of the icon images, including 'px'. Default= '0,0'. ]; } return $settings; } );
The following example shows how to use one SVG image for all five cluster image sizes. It also sets five custom ascending image sizes and text sizes, and sets the text color to white:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $settings ) { $clustericonsizes = array( 40, 45, 50, 55, 60 ); // specify custom icon image sizes $clustericontextsizes = array( 12, 14, 16, 18, 20 ); // specify custom icon text sizes for ( $i = 1; $i <= 5; $i ++ ) { $settings['config']['cluster']['styles'][] = [ 'url' => 'https://yourdomain/wp-content/themes/yourtheme/yourimages/clustericon.svg', // custom directory, required. The url becomes the CSS background-image url. 'width' => $clustericonsizes[ $i - 1 ], // required 'height' => $clustericonsizes[ $i - 1 ], // required 'textColor' => '#ffffff', // white, optional 'textSize' => $clustericontextsizes[ $i - 1 ], // optional // 'anchor' => [10,20], // Optional. Anchor position of the label text. By default label text is centered horizontally and vertically in the icon. [10,20] means 10px from the top of the icon (y) and 20px (x) from the left of the icon. Values need to be smaller than width and height of the icon to take effect. // 'iconAnchor' => [10,20], // Optional. The negative anchor position of the icon. [10,20] means the left top of the icon is 10px left of the lat/lng center, and 20px above the lat/lng center. Without iconAnchor values, the icon is centered on the lat/lng center horizontally and vertically. // 'backgroundPosition' => '5px 10px' // Optional. The CSS background-position of the icon images, including 'px'. Default= '0,0'. ]; } return $settings; } );
Method 3: Using cssClass
The third way of customizing cluster icons is by using the cssClass
option of the Gmaps Marker Clusterer library. The cluster “icons” in the code will then consist of a div
with the custom class you set, and will contain only the cluster icon text (the number of markers in the cluster):
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_filter( 'facetwp_map_init_args', function( $args ) { if ( isset( $args['config']['cluster'] ) ) { $args['config']['cluster']['cssClass'] = 'my-cluster-class'; } return $args; } );
You can then style the cluster icons in any way you like. Below is an example of accompanying CSS to style the
.my-cluster-class
div, resulting in a cluster icon looking like the image on the right.
Be aware that the position: absolute;
rule is not optional.
How to use custom CSS?
CSS code can be placed in your (child) theme's style.css file. Alternatively, you can add it manually between
<style>
tags in the<head>
section, in your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More info.my-cluster-class { position: absolute; /* not optional */ width: 36px; height: 36px; border-radius: 50%; background: #ff0000; border: 2px solid #fff; cursor: pointer; color: #fff; font-weight: bold; font-size: 14px; line-height: 32px; text-align: center; }
Customize marker cluster images by location or post id
The following example shows how to customize marker cluster images based on the location of the cluster, or the IDs of the posts it contains.
This example sets the class of a cluster icon to region1
if its lat
is larger than 38.0
.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'facetwp_scripts', function () { ?> <script> (function($) { document.addEventListener('facetwp-maps-loaded', function() { const observer = new MutationObserver((mutations) => { let clusters = FWP_MAP.mc.getClusters(); clusters.forEach(function(cluster) { let lat = cluster.getCenter().lat(); // latitude of cluster position let lng = cluster.getCenter().lng(); // longitude of cluster position // console.log(lat, lng); let markers = cluster.getMarkers(); // array of markers in this cluster let posts = markers.map((obj) => obj.post_id); // array of post ids from the markers in the cluster // console.log(posts); // Write conditions to set a cluster icon class, e.g. by lat and/or lng of the cluster. Or use the post IDs in the cluster. if (cluster.clusterIcon_ && cluster.clusterIcon_.div_ && lat > 38.0) { cluster.clusterIcon_.div_.className = 'region1'; // Use the class to set a background image for the cluster icon. } }); }); google.maps.event.addListener(FWP_MAP.map, 'idle', function() { const mapElement = document.getElementById('facetwp-map'); if (mapElement) { observer.observe(mapElement, { childList: true, subtree: true }); } }); }); })(fUtil); </script> <?php }, 100 );
The class can then be used to overwrite the CSS background-image
of the cluster:
How to use custom CSS?
CSS code can be placed in your (child) theme's style.css file. Alternatively, you can add it manually between
<style>
tags in the<head>
section, in your (child) theme's header.php file. You can also load it with a hook in your (child) theme's functions.php file, or in the Custom Hooks add-on. To load the code only on pages with facets, use thefacetwp_scripts
hook. To load it on all pages, usewp_head
orwp_footer
. Or you can use a code snippets plugin. More info.region1 { background-image: url("/path-to/region1.png") !important; }
Exclude the Proximity marker pin from marker clustering
If you are using the map with a Proximity facet, a yellow location marker is displayed on the map when a location is entered. This Proximity marker will be part of the closest marker cluster and will also be counted in the cluster’s displayed number.
To exclude the Proximity marker pin from the clustering behavior, add the following code to your (child) theme’s functions.php:
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
add_action( 'facetwp_scripts', function() { ?> <script type="text/javascript"> (function() { document.addEventListener('facetwp-loaded', function() { var markerClusterer = FWP_MAP.mc; var proximitymarker = markerClusterer.getMarkers().find(m => typeof m.post_id === 'undefined'); if (proximitymarker) { markerClusterer.removeMarker(proximitymarker); proximitymarker.setMap(FWP_MAP.map); } }); })(); </script> <?php }, 100 );
It is also possible to disable the Proximity marker pin entirely.
Customize cluster click behavior
If you want to customize the functionality of the map clusters, the following code shows how to access the marker cluster click event.
Two basic examples are shown: the first uses fitBounds()
to zoom into a cluster when it is clicked, which essentially does the same as setting zoomOnClick to true. The second example gets all markers in the clicked cluster, with the getMarkers()
method.
More methods that can be used with the Gmaps Marker Clusterer library can be found here.
How to use custom PHP code?
PHP code can be added to your (child) theme's functions.php file. Alternatively, you can use the Custom Hooks add-on, or a code snippets plugin. More info
<?php add_action( 'facetwp_scripts', function() { ?> <script type="text/javascript"> (function($) { ClusterIcon.prototype.triggerClusterClick = function() { var markerClusterer = this.cluster_.getMarkerClusterer(); // Trigger the clusterclick event google.maps.event.trigger(markerClusterer, 'clusterclick', this.cluster_); // Your code here. Two examples: // Example 1: Zoom in to bounds of cluster. Does the same as when zoomOnClick is set to true this.map_.fitBounds(this.cluster_.getBounds()); // Example 2: Put all markers in the clicked cluster into an object var markers = this.cluster_.getMarkers(); console.log(markers); } })(jQuery); </script> <?php }, 100 );